10个不稳定版本 (3个破坏性更新)
0.4.2 | 2024年5月20日 |
---|---|
0.4.1 | 2024年5月14日 |
0.4.0 | 2024年2月28日 |
0.3.0 | 2024年2月7日 |
0.1.3 | 2024年1月5日 |
在#derive-debug中排名第119
被3个框架使用(通过crabslab)
21KB
430 行
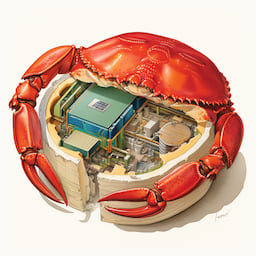
什么是
crabslab
是一个专注于在CPU和GPU之间打包数据的slab实现。
但是为什么?
以预期的形式将数据加载到GPU上很困难。
为了正确打包您的数据,您必须了解数据底层表示的对齐和大小。这通常会令您感到惊讶!
另一方面,使用slab,只需确保您的类型可以写入数组并从数组中读取。
观点
使用slab与着色器一起工作要容易得多。
可以使用rust-gpu
在Rust中编写着色器代码,这将使您能够在CPU和GPU代码上使用此crate。
rust-gpu
此crate是为了与rust-gpu
一起使用而制作的。具体来说,使用此crate,您可以将您的类型打包到CPU上的缓冲区中,然后从GPU上的slab(在Rust中)中读取您的类型。
其他no-std平台
尽管这个crate是为rust-gpu编写的,但它应该适用于其他no-std
环境。
以及如何
想法很简单 - crabslab
帮助您管理一堆连续的u32
(大致形式为Vec<u32>
)。类型实现SlabItem
特质,该特质将类型写入slab的索引作为连续的u32
,并且对称地读取它们。
crabslab
包括
- 一些特质
Slab
GrowableSlab
SlabItem
- 为您的类型提供
SlabItem
的derive宏 - 一些用于处理slab的新结构
Id
Array
Offset
- 一个辅助结构体
CpuSlab
,它包装实现了GrowableSlab
的任何内容 - 为
glam
类型派生SlabItem
的功能
示例
use crabslab::{CpuSlab, Slab, GrowableSlab, SlabItem, Id};
use glam::{Vec3, Vec4};
#[derive(Debug, Default, SlabItem, PartialEq)]
struct Light {
direction: Vec3,
color: Vec4,
inner_cutoff: f32,
outer_cutoff: f32,
is_on: bool
}
impl Light {
fn standard() -> Self {
Light {
direction: Vec3::NEG_Z, // pointing down
color: Vec4::ONE, // white
inner_cutoff: 0.5,
outer_cutoff: 2.6,
is_on: true
}
}
}
fn cpu_code() -> (Id<Light>, Vec<u32>) {
let light = Light::standard();
// Create a new slab on the CPU-side.
// Using CpuSlab make `append` unambiguous, as `Vec` has its own `append` function.
let mut slab = CpuSlab::new(vec![]);
let id = slab.append(&light);
(id, slab.into_inner())
}
fn shader_code(light_id: Id<Light>, slab: &[u32]) {
let light = slab.read(light_id);
assert_eq!(Light::standard(), light);
}
let (light_id, slab) = cpu_code();
// marshalling your data depends on which GPU library you are using...
shader_code(light_id, &slab);
依赖项
~270–720KB
~17K SLoC