25 个版本
0.10.1 | 2024年5月14日 |
---|---|
0.10.0 | 2024年3月15日 |
0.9.0 | 2023年10月12日 |
0.8.3 | 2023年7月31日 |
#63 in 图像
每月168次下载
在 oculante 中使用
4MB
892 行
lutgen-rs
为任意和流行的色彩方案提供闪电般的快速插值 LUT 生成器和应用器。将任何图像调整为您的桌面颜色方案!
示例输出
Hald Cluts
Catppuccin Mocha
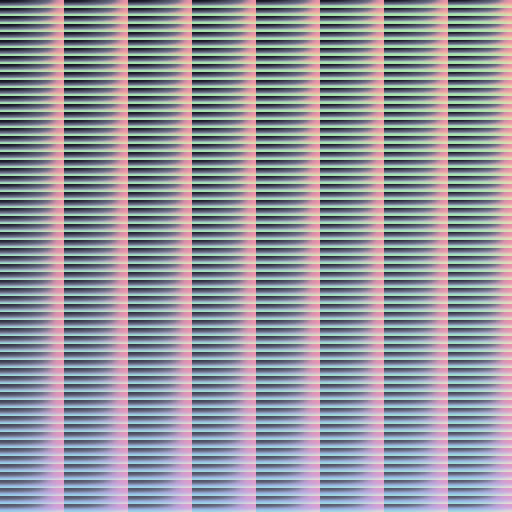
Gruvbox Dark
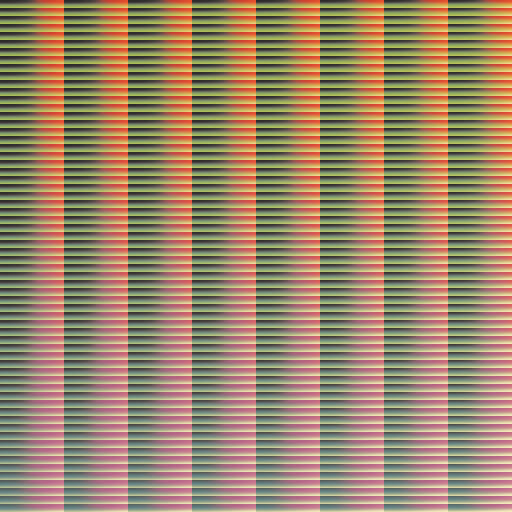
Nord
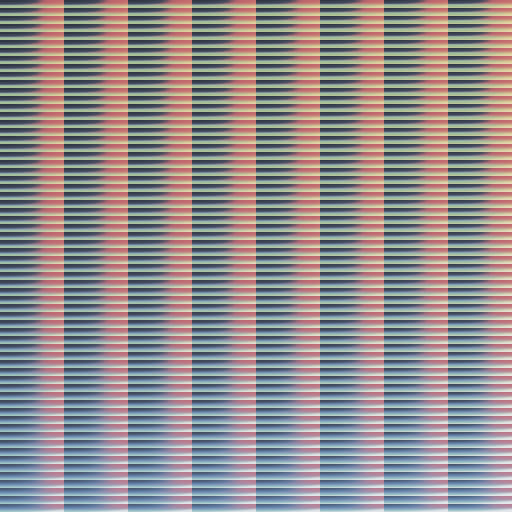
色彩调整
原始图像
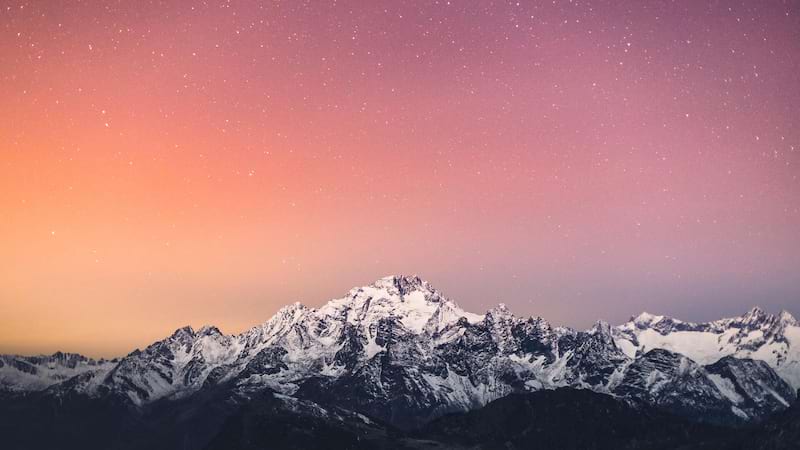
Catppuccin Mocha
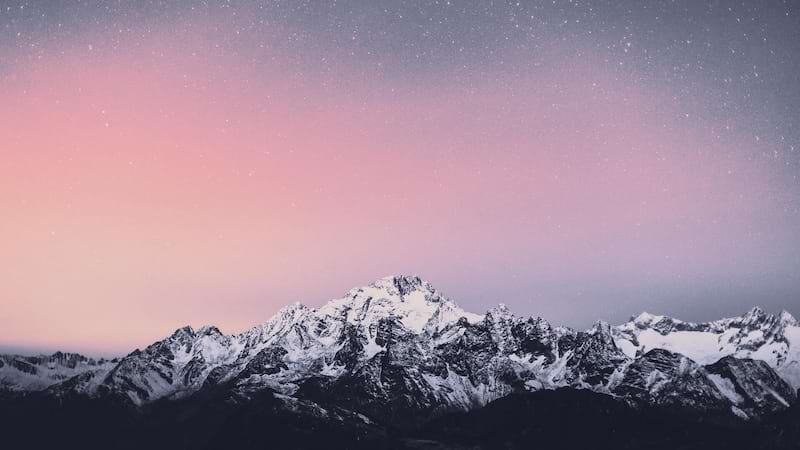
Gruvbox Dark
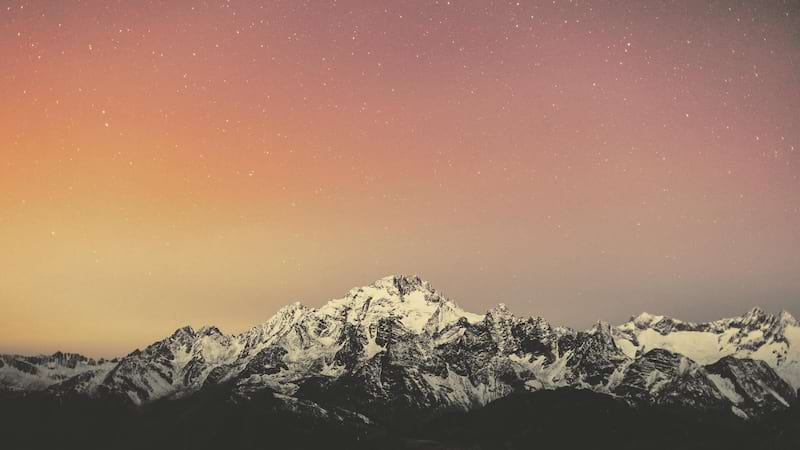
Nord
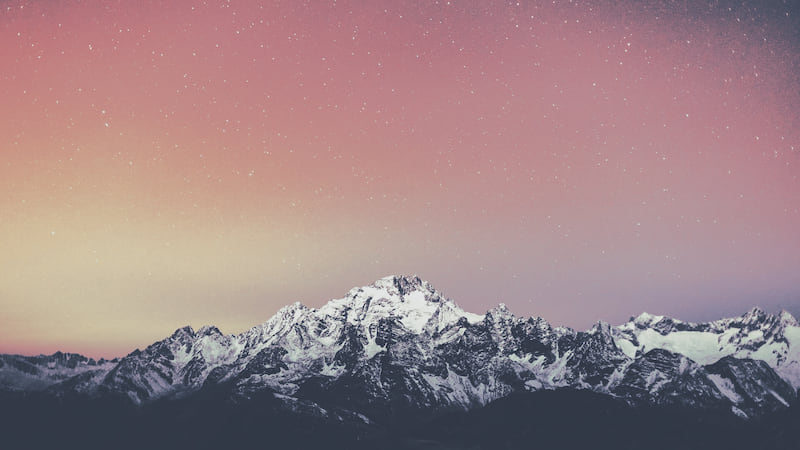
用法
注意:二进制和库用法相当稳定,但任何造成破坏性变更的版本都将升级到 0.X.0
命令行界面
源代码
git clone https://github.com/ozwaldorf/lutgen-rs
cd lutgen-rs
cargo install --path .
Nix flake
有可用的 nix flake,可以轻松运行
nix run github:ozwaldorf/lutgen-rs
通过 https://garnix.io 提供缓存
帮助文本
Usage: lutgen <COMMAND>
Commands:
generate Generate a hald clut for external or manual usage
apply Correct an image using a hald clut, either generating it, or loading it externally
completions Generate shell completions
help Print this message or the help of the given subcommand(s)
Options:
-h, --help Print help
-V, --version Print version
示例
校正图像
# Builtin palette
lutgen apply -p catppuccin-mocha docs/example-image.jpg -o mocha_version.jpg
# Custom colors
lutgen apply docs/example-image.jpg -- "#ABCDEF" ffffff 000000
# Custom palette file
lutgen apply docs/example-image.jpg -- $(cat palette.txt)
# Multiple images
lutgen apply image1.png image2.png *.jpg -p catppuccin-mocha
# Using an external LUT
lutgen apply --hald-clut mocha_lut.png docs/example-image.jpg
生成用于外部或手动使用的独立 LUT
# Builtin palette
lutgen generate -p catppuccin-mocha -o mocha_lut.png
# Custom colors
lutgen generate -o custom.png -- "#ABCDEF" ffffff 000000
# Custom palette file with hex codes
lutgen generate -o custom.png -- $(cat palette.txt)
校正视频(使用 ffmpeg)
ffmpeg -i input.mkv -i hald_clut.png -filter_complex '[0][1] haldclut' output.mp4
Zsh 完整性
lutgen completions zsh > _lutgen
sudo mv _lutgen /usr/local/share/zsh/site-functions/
库
默认情况下,启用了
bin
功能和依赖项。当用作库时,建议使用default-features = false
以最小化依赖关系树和构建时间。
生成 LUT(简单)
use lutgen::{
GenerateLut,
interpolation::{
GaussianRemapper, GaussianSamplingRemapper
},
};
use lutgen_palettes::Palette;
// Get a premade palette
let palette = Palette::CatppuccinMocha.get();
// Setup the fast Gaussian RBF algorithm
let (shape, nearest, lum_factor, preserve) = (128.0, 0, 1.0, false);
let remapper = GaussianRemapper::new(&palette, shape, nearest, lum_factor, preserve);
// Generate and remap a HALD:8 for the provided palette
let hald_clut = remapper.generate_lut(8);
// hald_clut.save("output.png").unwrap();
// Setup another palette to interpolate from, with custom colors
let palette = vec![
[255, 0, 0],
[0, 255, 0],
[0, 0, 255],
];
// Setup the slower Gaussian Sampling algorithm
let (mean, std_dev, iters, lum_factor, seed) = (0.0, 20.0, 512, 1.0, 420);
let remapper = GaussianSamplingRemapper::new(
&palette,
mean,
std_dev,
iters,
lum_factor,
seed
);
// Generate and remap a HALD:4 for the provided palette
let hald_clut = remapper.generate_lut(4);
// hald_clut.save("output.png").unwrap();
应用 LUT
use image::open;
use lutgen::{
identity::correct_image,
interpolation::GaussianRemapper,
GenerateLut,
};
use lutgen_palettes::Palette;
// Generate a hald clut
let palette = Palette::CatppuccinMocha.get();
let remapper = GaussianRemapper::new(&palette, 96.0, 0, 1.0, false);
let hald_clut = remapper.generate_lut(8);
// Save the LUT for later
hald_clut.save("docs/catppuccin-mocha-hald-clut.png").unwrap();
// Open an image to correct
let mut external_image = open("docs/example-image.jpg").unwrap().to_rgb8();
// Correct the image using the hald clut we generated
correct_image(&mut external_image, &hald_clut);
// Save the edited image
external_image.save("docs/catppuccin-mocha.jpg").unwrap()
直接重映射图像(高级)
注意:虽然重映射器可以直接在图像上使用,但使用 LUT 重映射并校正图像要快得多。
use lutgen::{
GenerateLut,
interpolation::{GaussianRemapper, InterpolatedRemapper},
};
// Setup the palette to interpolate from
let palette = vec![
[255, 0, 0],
[0, 255, 0],
[0, 0, 255],
];
// Setup a remapper
let (shape, nearest, lum_factor, preserve) = (96.0, 0, 1.0, false);
let remapper = GaussianRemapper::new(
&palette,
shape,
nearest,
lum_factor,
preserve
);
// Generate an image (generally an identity lut to use on other images)
let mut hald_clut = lutgen::identity::generate(8);
// Remap the image
remapper.remap_image(&mut hald_clut);
// hald_clut.save("output.png").unwrap();
计划中的功能
- 在低级 LUT(<16)校正时提供插值以提高精度
- 对图像应用 LUT 的硬件加速
源代码
- Hald Cluts: https://www.quelsolaar.com/technology/clut.html
- 使用 Hald Cluts 进行编辑: https://im.snibgo.com/edithald.htm
- 稀疏哈尔德聚类:https://im.snibgo.com/sphaldcl.htm
- RBF插值:https://en.wikipedia.org/wiki/Radial_basis_function_interpolation
- 谢泼德方法:https://en.wikipedia.org/wiki/Inverse_distance_weighting
- Oklab色彩空间:https://bottosson.github.io/posts/oklab/
特别感谢
- Stonks3141 维护 Alpine Linux 包
星史
依赖
~7–34MB
~526K SLoC