6 个版本 (重大更新)
新版本 0.5.0 | 2024 年 8 月 13 日 |
---|---|
0.4.0 | 2024 年 6 月 7 日 |
0.3.0 | 2024 年 5 月 19 日 |
0.2.0 | 2024 年 5 月 9 日 |
0.1.1 | 2024 年 4 月 30 日 |
#210 in 图像
每月下载 129 次
用于 2 crate
225KB
5.5K SLoC
auto-palette
🎨 一个用于自动从图像中提取突出色彩调板的 Rust 库。
特性
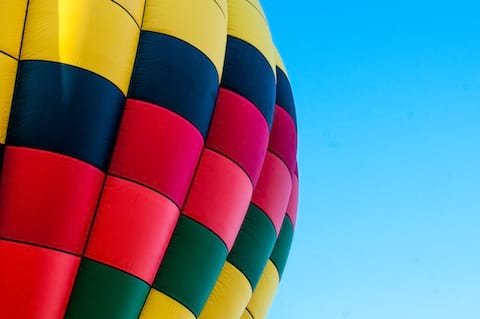

[!NOTE] 图片由 Laura Clugston 在 Unsplash 提供
- 自动从图像中提取突出色彩调板。
- 提供关于颜色、位置和数量的详细信息。
- 支持多种提取算法,包括
DBSCAN
、DBSCAN++
和KMeans++
。 - 支持多种色彩空间,包括
RGB
、HSL
和LAB
。 - 支持基于多种主题选择突出颜色,包括
Vivid
、Muted
、Light
和Dark
。
安装
在 Rust 项目中使用 auto-palette
,将其添加到您的 Cargo.toml
文件中。
[dependencies]
auto-palette = "0.5.0"
用法
以下是一个基本示例,演示了如何提取调板并找到突出颜色。更多示例请参阅 示例目录。
use auto_palette::{ImageData, Palette};
fn main() {
// Load the image data from the file
let image_data = ImageData::load("../../gfx/holly-booth-hLZWGXy5akM-unsplash.jpg").unwrap();
// Extract the color palette from the image data
let palette: Palette<f64> = Palette::extract(&image_data).unwrap();
println!("Extracted {} swatches", palette.len());
// Find the 5 prominent colors in the palette and print their information
let swatches = palette.find_swatches(5);
for swatch in swatches {
println!("Color: {}", swatch.color().to_hex_string());
println!("Position: {:?}", swatch.position());
println!("Population: {}", swatch.population());
}
}
API
有关 API 的更多信息,请参阅 文档。
ImageData
ImageData
结构体表示用于提取色彩调板的图像数据。
ImageData::load
从文件中加载图像数据。
支持的图像格式包括 PNG
、JPEG
、GIF
、BMP
、TIFF
和 WEBP
。
此方法需要启用 image
功能。默认情况下,image
功能已启用。
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
ImageData::新版本
从原始图像数据创建一个新的实例。
每个像素由四个连续的字节表示,顺序为 R
、G
、B
和 A
。
// Create a new instance from the raw image data
let pixels = [
255, 0, 0, 255, // Red
0, 255, 0, 255, // Green
0, 0, 255, 255, // Blue
255, 255, 0, 255, // Yellow
];
let image_data = ImageData::new(2, 2, &pixels).unwrap();
Palette
Palette
结构体表示从 ImageData
中提取的颜色调色板。
Palette::extract
Palette::extract_with_algorithm
Palette::find_swatches
Palette::find_swatches_with_theme
Palette::extract
从给定的 ImageData
中提取颜色调色板。此方法用于使用默认的 Algorithm
(DBSCAN)提取颜色调色板。
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
// Extract the color palette from the image data
let palette: Palette<f64> = Palette::extract(&image_data).unwrap();
Palette::extract_with_algorithm
使用指定的 Algorithm
从给定的 ImageData
中提取颜色调色板。支持以下算法:DBSCAN
、DBSCAN++
和 KMeans++
。
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
// Extract the color palette from the image data with the specified algorithm
let palette: Palette<f64> = Palette::extract_with_algorithm(&image_data, Algorithm::DBSCAN).unwrap();
Palette::find_swatches
根据色卡数量查找调色板中的突出颜色。
返回的色卡按其数量降序排序。
// Find the 5 prominent colors in the palette
let swatches = palette.find_swatches(5);
Palette::find_swatches_with_theme
根据指定的 Theme
和色卡数量查找调色板中的突出颜色。支持的主题有 Basic
、Colorful
、Vivid
、Muted
、Light
和 Dark
。
// Find the 5 prominent colors in the palette with the specified theme
let swatches = palette.find_swatches_with_theme(5, Theme::Light);
Swatch
Swatch
结构体表示 Palette
中的颜色色卡。
它包含有关颜色、位置、数量和比率的详细信息。
// Find the 5 prominent colors in the palette
let swatches = palette.find_swatches(5);
for swatch in swatches {
// Get the color, position, and population of the swatch
println!("Color: {:?}", swatch.color());
println!("Position: {:?}", swatch.position());
println!("Population: {}", swatch.population());
println!("Ratio: {}", swatch.ratio());
}
[!TIP]
Color
结构体提供了将颜色转换为不同格式的方法,例如RGB
、HSL
和CIE L*a*b*
。let color = swatch.color(); println!("Hex: {}", color.to_hex_string()); println!("RGB: {:?}", color.to_rgb()); println!("HSL: {:?}", color.to_hsl()); println!("CIE L*a*b*: {:?}", color.to_lab()); println!("Oklch: {:?}", color.to_oklch());
开发
按照以下说明构建和测试项目
- 分叉并克隆仓库。
- 为您的功能或错误修复创建一个新的分支。
- 进行更改并编写测试。
- 使用
cargo test --lib
测试您的更改。 - 使用
cargo +nightly fmt
和taplo fmt
格式化代码。 - 创建一个拉取请求。
许可
本项目根据 MIT 许可证分发。有关详细信息,请参阅 LICENSE 文件。
依赖项
~5.5MB
~106K SLoC