6个版本
0.1.5 | 2023年4月24日 |
---|---|
0.1.4 | 2023年4月22日 |
#253 在 图形API 中
67 每月下载量
24KB
653 代码行
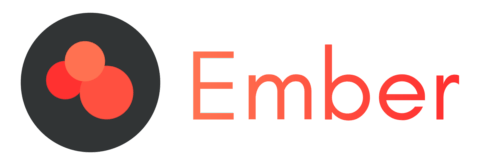
Ember
Ember是一个简单的Rust 2D渲染库,允许您轻松地创建图形和交互式应用程序。它使用minifb
crate来处理窗口创建和输入事件。
特性
- 用于绘制基本形状(如圆形、线条和矩形)的简单易用的API
- 支持使用内置字体绘制文本
- 高效的像素缓冲区操作
- 鼠标和键盘输入处理
示例
基本绘图示例
以下示例展示了Ember的一些特性
use ember_rs::Ember;
fn main() {
let (width, height, fps) = (800, 600, 144.0);
let mut app = Ember::new("Ember - Simple Example", width, height, fps);
while !app.should_close() {
// clear the previous drawing
app.clear();
// draw text at the top-left of the screen at size 4
app.draw_text("ember is awesome", 20, 20, 4, 0xFFAAAA);
// draw a filled white circle at the bottom-right of the window
app.draw_circle_fill(700, 500, 40, 0xFFFFFF);
// draw a green-ish line going from the text to the circle
app.draw_line(310, 70, 630, 430, 0xAAFFAA);
// update the screen with the new drawing
app.update();
}
}
鼠标和键盘输入示例
以下示例展示了鼠标和键盘输入的处理
use ember_rs::{Ember, Key};
fn main() {
let (width, height, fps) = (800, 600, 144.0);
let mut app = Ember::new("Ember - Mouse and Keyboard Example", width, height, fps);
let mut circle_x = width / 2;
let mut circle_y = height / 2;
let circle_radius = 40;
while !app.should_close() {
// clear the previous drawing
app.clear();
// handle keyboard input
app.process_keys(|key| match key {
Key::W => circle_y -= 5,
Key::A => circle_x -= 5,
Key::S => circle_y += 5,
Key::D => circle_x += 5,
_ => {}
});
// alternatively use app.get_keys() which returns a Vec<Key>
// handle mouse input
let mouse_info = app.get_mouse_info();
if let Some((mouse_x, mouse_y)) = mouse_info.position {
if mouse_info.left_button {
circle_x = mouse_x as i32;
circle_y = mouse_y as i32;
}
}
// draw a filled circle at the current position
app.draw_circle_fill(circle_x, circle_y, circle_radius, 0xFF0000);
// update the screen with the new drawing
app.update();
}
}
填充形状示例
以下示例展示了绘制各种填充形状
// this method is used under the hood for all the other methods
app.set_pixel(x, y, color);
// draw a line
app.draw_line(x1, y1, x2, y2, color);
// draw a line with a specific width
app.draw_line_width(x1, y1, x2, y2, width, color);
// draw a rectangle
app.draw_rectangle(x, y, width, height, color);
// draw a filled rectangle
app.draw_rectangle_fill(x, y, width, height, color);
// draw a circle
app.draw_circle(x, y, radius, color);
// draw a filled circle
app.draw_circle_fill(x, y, radius, color);
绘制文本示例
以下示例展示了如何在屏幕上绘制文本
use ember_rs::{Ember, helper};
fn main() {
let (width, height, fps) = (800, 600, 144.0);
let mut app = Ember::new("Ember - Mouse and Keyboard Example", width, height, fps);
while !app.should_close() {
// clear the previous drawing
app.clear();
// draw text to the screen at position (20, 20), scaled 4 times
app.draw_text("hello world", 50, 50, 4, 0xFFFFFF);
// if you want to center the text, use one of the helper methods
let text = format!("Width: {}", width);
// this return the (x, y) needed to center the text
let (x, y) = helper::center_text(&text, 400, 400, 4);
// draw the centered text
app.draw_text(&text, x, y, 4, 0xAAAAFF);
// update the screen with the new drawing
app.update();
}
}
安装
要开始使用Ember,请在您的项目中输入以下命令
cargo add ember-rs
依赖关系
~0.4–2.5MB
~43K SLoC