46个版本
0.3.15 | 2022年12月24日 |
---|---|
0.3.14 | 2022年12月14日 |
0.3.1 | 2022年11月13日 |
0.2.21 | 2022年11月8日 |
0.1.11 | 2022年3月28日 |
#207 in 渲染
每月152次下载
1MB
7.5K SLoC
Bevy的实时路径追踪器
bevy-hikari
是 Bevy 的全局照明的实现。
Bevy发布0.8版本后,插件转移到延迟混合路径追踪。对于旧版本(0.1.x),它使用具有各向异性米波映射的体素锥追踪,请查看 bevy-0.6
分支。
🚧 此项目正在积极开发中。某些过程尚未优化,公共接口可能在主要版本之间发生变化,并且可能非常频繁地发布小版本。
⚠️ 注意:
- 请使用
--release
标志运行示例,以避免纹理非均匀索引错误 - 当作为依赖项使用时,请以 发布模式 编译此库
- 支持的网格必须具有这3个顶点属性:位置、法线和uv
Bevy版本支持
bevy |
bevy-hikari |
---|---|
0.6 | 0.1 |
0.8 | 0.2 |
0.9 | 0.3 |
进度
- 网格资源和实例的提取和准备
- 异步构建加速结构
- 生成G-缓冲区
- N次间接照明
- 透明度
- 下一事件估计
- 更好的光线采样(L-BVH + Alias表)
- ReSTIR:时间样本重用
- ReSTIR:空间样本重用
- 时空滤波器
- 时间反走样
- 空间上采样(FSR 1.0)
- 时间上采样(SMAA TU4X)
- 骨骼动画
- HDR输出
- 辉光
- 硬件光线追踪(上游相关)
基本用法
- 在
App
中添加HikariPlugin
后添加PbrPlugin
- 使用方向光设置场景
- 将
HikariSettings
组件插入到相机中
可以通过相机实体上的 HikariSettings
组件配置渲染器。可用的选项包括
pub struct HikariSettings {
/// The interval of frames between sample validation passes.
pub direct_validate_interval: usize,
/// The interval of frames between sample validation passes.
pub emissive_validate_interval: usize,
/// Temporal reservoir sample count is capped by this value.
pub max_temporal_reuse_count: usize,
/// Spatial reservoir sample count is capped by this value.
pub max_spatial_reuse_count: usize,
/// Max lifetime of a reservoir sample before being replaced with new one.
pub max_reservoir_lifetime: f32,
/// Half angle of the solar cone apex in radians.
pub solar_angle: f32,
/// Count of indirect bounces.
pub indirect_bounces: usize,
/// Threshold for the indirect luminance to reduce fireflies.
pub max_indirect_luminance: f32,
/// Clear color override.
pub clear_color: Color,
/// Whether to do temporal sample reuse in ReSTIR.
pub temporal_reuse: bool,
/// Whether to do spatial sample reuse for emissive lighting in ReSTIR.
pub emissive_spatial_reuse: bool,
/// Whether to do spatial sample reuse for indirect lighting in ReSTIR.
pub indirect_spatial_reuse: bool,
/// Whether to do noise filtering.
pub denoise: bool,
/// Which temporal filtering implementation to use.
pub taa: Taa,
/// Which upscaling implementation to use.
pub upscale: Upscale,
}
如果您对这些选项中的某些术语不确定,您可以查看 渲染过程 以获取详细信息。
use bevy::prelude::*;
use bevy_hikari::prelude::*;
use std::f32::consts::PI;
fn main() {
App::new()
.add_plugins(DefaultPlugins)
.add_plugin(HikariPlugin {
remove_main_pass: true,
})
.add_startup_system(setup)
.run();
}
fn setup(
mut commands: Commands,
mut meshes: ResMut<Assets<Mesh>>,
mut materials: ResMut<Assets<StandardMaterial>>,
_asset_server: Res<AssetServer>,
) {
// Plane
commands.spawn(PbrBundle {
mesh: meshes.add(Mesh::from(shape::Plane { size: 5.0 })),
material: materials.add(Color::rgb(0.3, 0.5, 0.3).into()),
..default()
});
// Cube
commands.spawn(PbrBundle {
mesh: meshes.add(Mesh::from(shape::Cube { size: 1.0 })),
material: materials.add(Color::rgb(0.8, 0.7, 0.6).into()),
transform: Transform::from_xyz(0.0, 0.5, 0.0),
..default()
});
// Only directional light is supported
commands.spawn(DirectionalLightBundle {
directional_light: DirectionalLight {
illuminance: 10000.0,
..Default::default()
},
transform: Transform {
translation: Vec3::new(0.0, 5.0, 0.0),
rotation: Quat::from_euler(EulerRot::XYZ, -PI / 4.0, PI / 4.0, 0.0),
..Default::default()
},
..Default::default()
});
// Camera
commands.spawn((
Camera3dBundle {
// Set `camera_render_graph`
camera_render_graph: CameraRenderGraph::new(bevy_hikari::graph::NAME),
transform: Transform::from_xyz(-2.0, 2.5, 5.0).looking_at(Vec3::ZERO, Vec3::Y),
..Default::default()
},
// Add [`HikariSettings`] component to enable GI rendering
HikariSettings::default(),
));
}
自定义材料
bevy-hikari
是基于 bevy
的 PBR 标准材质构建的。一个有资格进行光照的材质需要具有与 StandardMaterial
相同的表面属性(基础颜色、金属度等)。为了使用自定义材质进行渲染,
- 为材质
CustomMaterial
实现Into<StandardMaterial>
,并且 - 将
GenericMaterialPlugin::<CustomMaterial>::default()
添加到应用中 - 将
GenericInstancePlugin::<CustomMaterial>::default()
添加到应用中
屏幕截图
以下是示例的截图。
简单
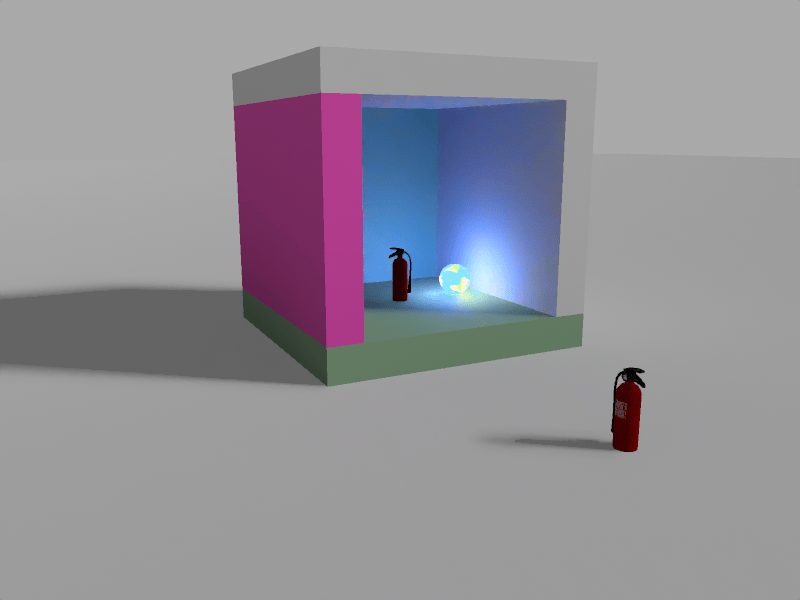
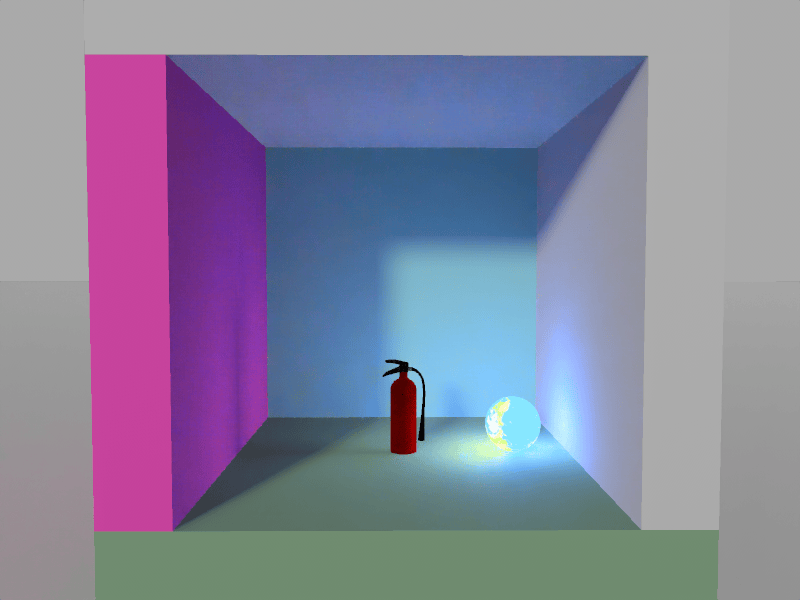
康奈尔(2次间接反弹)
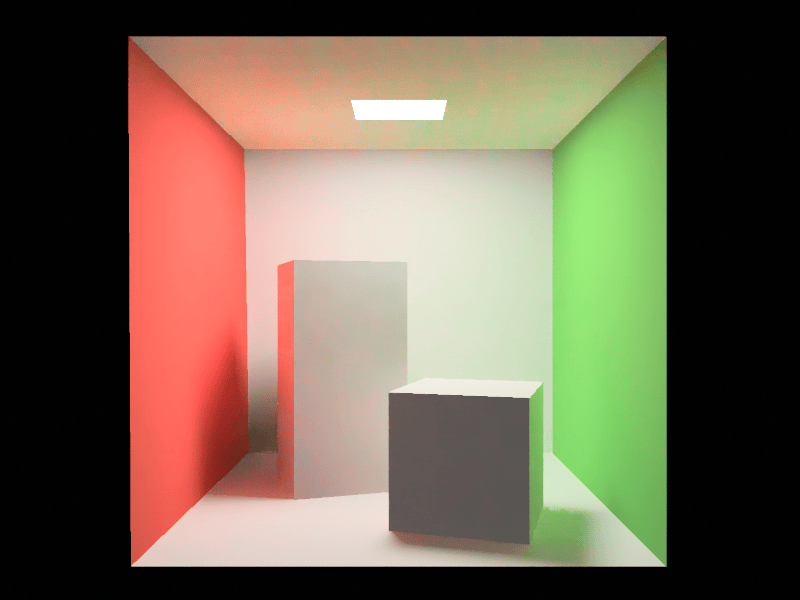
城市(宋代)
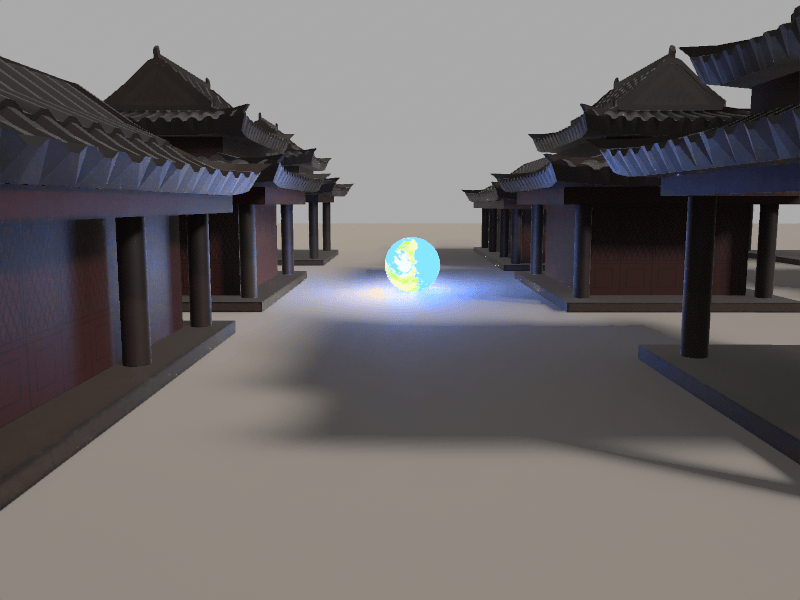
场景(二战)
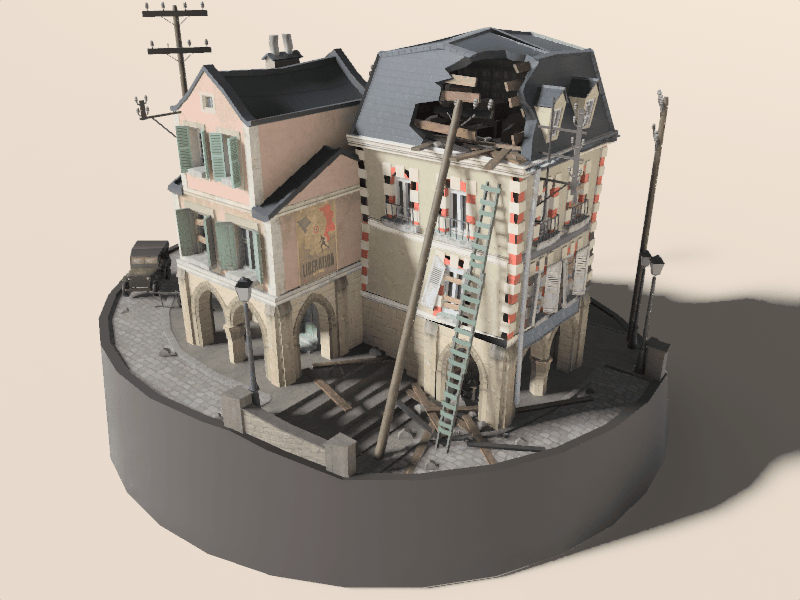
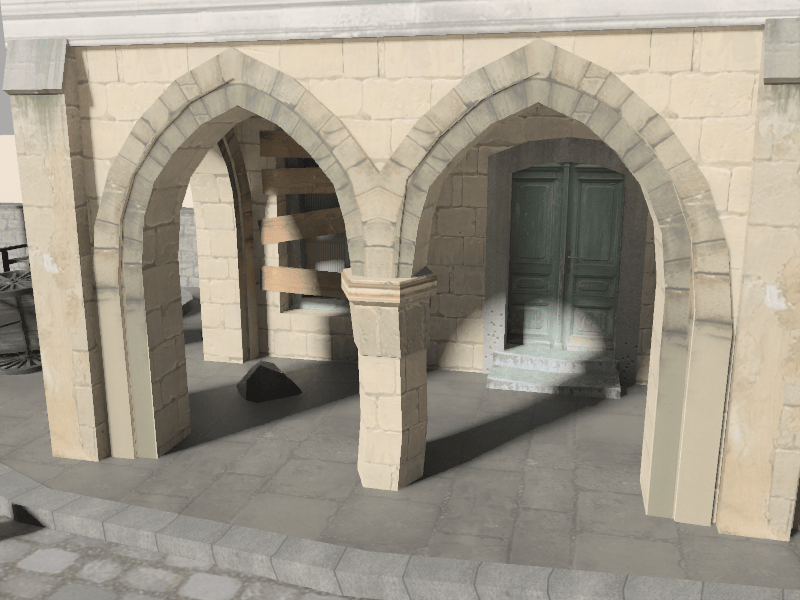
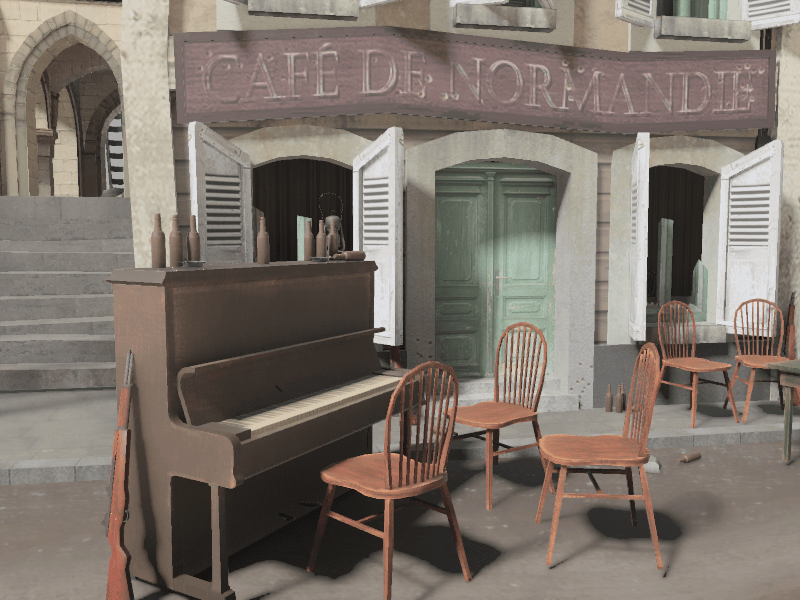
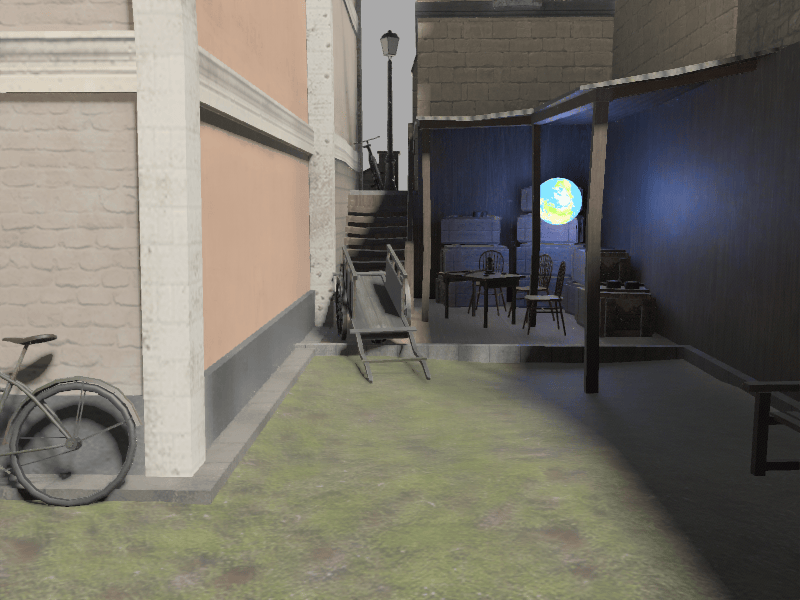
渲染通道
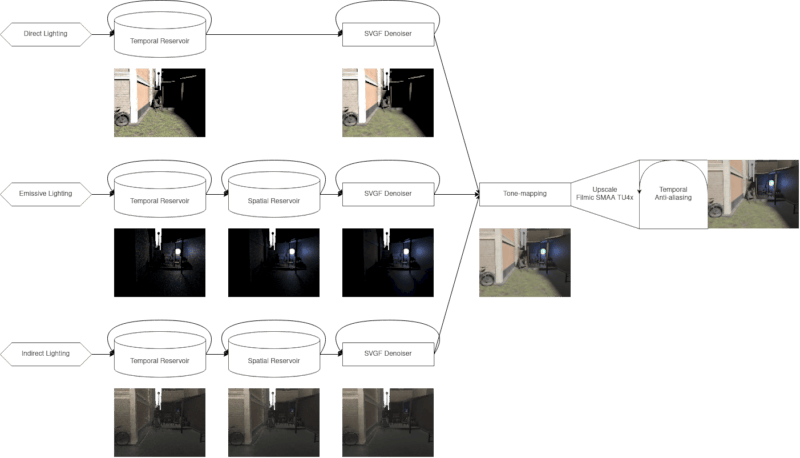
许可证
就像 Bevy 一样,这个存储库中的所有代码都可以根据您的选择在以下两种许可证下获得双授权:
- MIT 许可证 (LICENSE-MIT 或 https://open-source.org.cn/licenses/MIT)
- Apache License,版本 2.0 (LICENSE-APACHE 或 https://apache.ac.cn/licenses/LICENSE-2.0)
任选其一。
致谢
- "灭火器" 模型和纹理由 Cameron 'cron' Fraser 提供。
- "二战城市场景" 来自 sketchfab。
- "宋代建筑" 由 唐晨 提供。
参考
- ReSTIR: https://cs.dartmouth.edu/wjarosz/publications/bitterli20spatiotemporal.pdf
- ReSTIR GI: https://d1qx31qr3h6wln.cloudfront.net/publications/ReSTIR%20GI.pdf
- GRIS: https://d1qx31qr3h6wln.cloudfront.net/publications/sig22_GRIS.pdf
- SVGF: https://cg.ivd.kit.edu/publications/2017/svgf/svgf_preprint.pdf
- Filmic SMAA TU: https://www.activision.com/cdn/research/Dynamic_Temporal_Antialiasing_and_Upsampling_in_Call_of_Duty_v4.pdf
依赖项
~38–53MB
~733K SLoC