16个版本 (4个重大更新)
0.5.0 | 2023年10月14日 |
---|---|
0.4.12 | 2023年5月28日 |
0.4.5 | 2023年4月25日 |
0.4.4 | 2023年3月12日 |
0.1.0 | 2023年2月9日 |
#183 in 测试
每月88次下载
1MB
24K SLoC
SAPTest
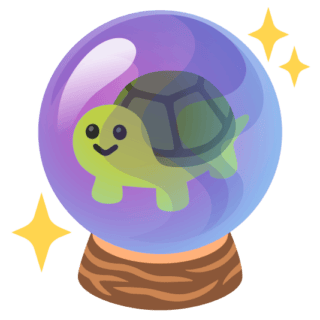
Super Auto Pets的数据库和测试框架。
游戏信息从Super Auto Pets维基页面查询并存储在SQLite
数据库中。
用法
使用cargo
将其添加到项目中。
cargo add saptest
团队
构建一个Team
并在它们之间模拟战斗。
然后在.dot
格式中可视化结果!
use saptest::{
Pet, PetName, Food, FoodName,
Team, TeamCombat, Position, create_battle_digraph
};
// Create a team.
let mut team = Team::new(
&vec![Some(Pet::try_from(PetName::Ant).unwrap()); 5],
5
).unwrap();
let mut enemy_team = team.clone();
// Set a seed for a team.
team.set_seed(Some(25));
// Give food to pets.
team.set_item(&Position::First, Food::try_from(FoodName::Garlic).ok()).unwrap();
enemy_team.set_item(&Position::First, Food::try_from(FoodName::Garlic).ok()).unwrap();
// And fight!
team.fight(&mut enemy_team).unwrap();
// Create a graph of the fight.
println!("{}", create_battle_digraph(&team, false));
digraph {
rankdir=LR
node [shape=box, style="rounded, filled", fontname="Arial"]
edge [fontname="Arial"]
0 [ label = "Ant_0 - The Fragile Truckers_copy" ]
1 [ label = "Ant_0 - The Fragile Truckers", fillcolor = "yellow" ]
2 [ label = "Ant_3 - The Fragile Truckers", fillcolor = "yellow" ]
3 [ label = "Ant_4 - The Fragile Truckers_copy" ]
0 -> 1 [ label = "(Attack, Damage (0, 2), Phase: 1)" ]
1 -> 0 [ label = "(Attack, Damage (0, 2), Phase: 1)" ]
1 -> 2 [ label = "(Faint, Add (1, 1), Phase: 1)" ]
0 -> 3 [ label = "(Faint, Add (1, 1), Phase: 1)" ]
}
图表可以像上面的例子一样简单...或者极其复杂。
商店
向Team
添加商店功能,掷骰子、冻结、购买/出售宠物和食物。
use saptest::{
Shop, ShopItem, TeamShopping, Team,
Position, Entity, EntityName, Food, FoodName,
db::pack::Pack
};
// All teams are constructed with a shop at tier 1.
let mut team = Team::default();
// All shop functionality is supported.
team.set_shop_seed(Some(1212))
.set_shop_packs(&[Pack::Turtle])
.open_shop().unwrap()
.buy(
&Position::First, // From first.
&Entity::Pet, // Pet
&Position::First // To first position, merging if possible.
).unwrap()
.move_pets(
&Position::First, // First pet.
&Position::Relative(-2), // To pet 2 slots behind. Otherwise, ignore.
true // And merge them if possible.
).unwrap()
.sell(&Position::First).unwrap()
.freeze_shop(&Position::Last, &Entity::Pet).unwrap()
.roll_shop().unwrap()
.close_shop().unwrap();
// Shops can be built separately and can replace a team's shop.
let mut tier_5_shop = Shop::new(3, Some(42)).unwrap();
let weakness = ShopItem::new(
Food::try_from(FoodName::Weak).unwrap()
);
tier_5_shop.add_item(weakness).unwrap();
team.replace_shop(tier_5_shop).unwrap();
宠物
构建自定义Pet
和Effect
。
use saptest::{
Pet, PetName, PetCombat,
Food, FoodName,
Entity, Position, Effect, Statistics,
effects::{
trigger::TRIGGER_START_BATTLE,
actions::GainType,
state::Target,
actions::Action
}
};
// Create known pets.
let mut pet = Pet::try_from(PetName::Ant).unwrap();
// Or custom pets and effects.
let custom_effect = Effect::new(
TRIGGER_START_BATTLE, // Effect trigger
Target::Friend, // Target
Position::Adjacent, // Positions
Action::Gain(GainType::DefaultItem(FoodName::Melon)), // Action
Some(1), // Number of uses.
false, // Is temporary.
);
let mut custom_pet = Pet::custom(
"MelonBear",
Statistics::new(50, 50).unwrap(),
&[custom_effect],
);
// Fight two pets individually as well.
// Note: Effects don't activate here.
pet.attack(&mut custom_pet);
日志记录
使用类似simple_logger
的crate启用日志记录。
配置
要配置全局SapDB
的启动,在项目的根目录中创建一个.saptest.toml
文件。
- 指定要查询的宠物、食物和令牌的页面版本。
- 在启动时切换重复更新的选项。
- 设置数据库文件名。
[database]
# https://superautopets.wiki.gg/index.php?title=Pets&oldid=4634
pets_version = 4634
filename = "./sap.db"
update_on_startup = false
在[general]
中使用build_graph
禁用图形构建。
[general]
build_graph = false
基准测试
saptest
的基准测试位于benches/battle_benchmarks.rs
中,并使用criterion
crate运行。
- 与
sapai
相比,这是一个用Python编写的Super Auto Pets测试框架。 - 两个测试都在AMD Ryzen 5 5600 6核处理器 @ 3.50 GHz上运行。
# saptest
git clone git@github.com:koisland/SuperAutoTest.git --depth 1
cargo add cargo-tarpaulin
cargo bench && open target/criterion/sapai_example/report/index.html
# sapai
cd benches/
git clone https://github.com/manny405/sapai.git && cd sapai
python setup.py install
# Then run `battle_benchmarks_sapai.ipynb`.
saptest(无图形)
- 待办事项
saptest(图形)
- 待办事项
sapai
- 每次循环4.29 ms ± 51.8 µs(7次运行的平均值 ± 标准差,每次100个循环)
待办事项
- 添加随机生成队伍的特性。
- 添加遍历记录字段/字段名的方法,并构建宏来构建SQL语句。
- 手动添加字段既繁琐又容易出错。
- 构建解析原始效果文本到
Effect
结构的词法分析器。 - 为每个包添加功能标志。
- 改进界面。
- 具有类似于
AsRef
和Into/TryInto
的函数参数。例如:Shop.add_item
- 使用
Shop<Open/Close>
的类型状态模式
- 具有类似于
来源
依赖项
~37MB
~629K SLoC