10 个版本
0.3.0 | 2022 年 12 月 1 日 |
---|---|
0.2.2 | 2022 年 8 月 3 日 |
0.2.1 | 2022 年 7 月 7 日 |
0.2.0 | 2021 年 8 月 1 日 |
0.0.0 | 2020 年 9 月 1 日 |
#98 in 电子邮件
84 每月下载
84KB
597 行
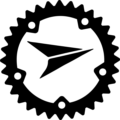
mailjet-rs
为 Rust 编写的 Mailjet API 包装器
概述
此软件包包含 Mailjet API 的非官方包装器。
官方资源可在 Mailjet 开发者指南 网站上找到。
官方的 GitHub 上的 Mailjet 组织 为 Go、PHP、JavaScript (NodeJS)、Ruby 等其他编程语言提供了包装器。
内容
安装
mailjet-rs = "0.2.0"
# Used by `Hyper` which is the HTTP request solution behind the Client
tokio = { version = "1", features = ["full"] }
客户端
Client
结构体执行与 API 相关的任务,例如处理身份验证和定义每个请求必须使用的 API 版本。
身份验证
Mailjet 的电子邮件 API 使用 Mailjet 为您的帐户提供的 API 密钥 此处。
这些用于创建 Client
实例,如下所示
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
API 版本
Mailjet 的 API 目前有 3 个版本可用,以下表格描述了每个版本及其在此软件包中的支持情况
版本 | 名称 | 支持 |
---|---|---|
v3 | 电子邮件 API | ✔️ |
v3.1 | 电子邮件发送 API v3.1(最新版本) | ❌(早期开发) |
v4 | 短信 API | ❌(待定) |
如您所见,目前此软件包仅支持电子邮件 API 的第 3 版。第 3.1 版的支持处于早期开发阶段
要使用的 API 版本通过 SendAPIVersion
枚举提供给 Client
。
发送消息
要发送一个 Message
,您必须创建一个 Client
实例,然后定义 Recipients
,最后构建您的 Message
。
Client
的方法 send
接收一个 Payload
特征实现者,这个特征由 Message
实现,以及通过 Client
发送到 Mailjet API 的每个结构。
对 send
的调用将返回一个 Future
,该 Future
包装了一个 Result<MailjetResponse, MailjetError>
。
示例要求
要运行以下任何示例,您必须拥有 Mailjet 的公钥和私钥(免费计划可用于使用 API),并且还需要安装 tokio 运行时。
mailjet-rs = "0.2.0"
# Used by `Hyper` which is the HTTP request solution behind the Client
tokio = { version = "1", features = ["full"] }
使用 API 包装器
消费此包装器有两种方式,要么使用由 Message
结构提供的方法,要么不使用这些方法。
Message
结构的所有字段都是公开的,这是因为有时通过调用多个方法构建一个简单的结构体可能有点冗长/繁琐。
提供的方法旨在根据 API 规范验证 Message
结构的字段,但您可以自由地为这些字段提供值。
基本消息
mailjet-rs 使用 Tokio 运行时执行异步操作,底层使用 Hyper 执行对 Mailjet API 的 HTTP 请求。
这里创建了一个 Message
并将其发送到定义的 Recipient
。此消息既不包含 HTML 也不使用模板,而是包含原始文本。
use mailjet_rs::common::Recipient;
use mailjet_rs::v3::Message;
use mailjet_rs::{Client, SendAPIVersion};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
// Create an instance of the Mailjet API client
// used to send the `Message` and also define your API
// credentials
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
// Create your a `Message` instance with the minimum required values
let mut message = Message::new(
"mailjet_sender@company.com",
"Mailjet Rust",
Some("Your email flight plan!".to_string()),
Some("Dear passenger, welcome to Mailjet! May the delivery force be with you!".to_string())
);
message.push_recipient(Recipient::new("receiver@company.com"));
// Finally send the message using the `Client`
let response = client.send(message).await;
// Do something with the response from Mailjet
// Ok(Response { sent: [Sent { email: "your_receiver@company.com", message_id: 000, message_uuid: "message-uuid" }] })
println!("{:?}", response);
Ok(())
}
发送到多个收件人
use mailjet_rs::common::Recipient;
use mailjet_rs::v3::Message;
use mailjet_rs::{Client, SendAPIVersion};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
let mut message = Message::new(
"mailjet_sender@company.com",
"Mailjet Rust",
Some("Your email flight plan!".to_string()),
Some("Dear passenger, welcome to Mailjet! May the delivery force be with you!".to_string())
);
let recipients = vec![
Recipient::new("receiver1@company.com"),
Recipient::new("receiver2@company.com"),
Recipient::new("receiver3@company.com"),
];
message.push_many_recipients(recipients);
let response = client.send(message).await;
println!("{:?}", response);
Ok(())
}
使用 To
、Cc
和 Bcc
而不是 收件人
注意:如果收件人不在您的任何联系列表中,它将从头创建,如果您计划发送欢迎邮件然后尝试将电子邮件添加到列表中(因为联系人实际上已经存在),请记住这一点。Mailjet 的 API 文档
use mailjet_rs::common::Recipient;
use mailjet_rs::v3::Message;
use mailjet_rs::{Client, SendAPIVersion};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
let mut message = Message::new(
"mailjet_sender@company.com",
"Mailjet Rust",
Some("Your email flight plan!".to_string()),
Some("Dear passenger, welcome to Mailjet! May the delivery force be with you!".to_string())
);
message.set_receivers(
vec![
Recipient::new("bar@foo.com"),
],
Some(vec![
Recipient::new("bee@foo.com"),
]),
None
);
let response = client.send(message).await;
println!("{:?}", response);
Ok(())
}
发送内联附件
use mailjet_rs::common::Recipient;
use mailjet_rs::v3::{Message, Attachment};
use mailjet_rs::{Client, SendAPIVersion};
/// Base64 representation of the Mailjet logo found in the Mailjet SendAPI V3 docs
const MAILJET_LOGO_BASE64: &str = "iVBORw0KGgoAAAANSUhEUgAAABQAAAALCAYAAAB/Ca1DAAAACXBIWXMAAA7EAAAOxAGVKw4bAAAAB3RJTUUH4wIIChcxurq5eQAAAAd0RVh0QXV0aG9yAKmuzEgAAAAMdEVYdERlc2NyaXB0aW9uABMJISMAAAAKdEVYdENvcHlyaWdodACsD8w6AAAADnRFWHRDcmVhdGlvbiB0aW1lADX3DwkAAAAJdEVYdFNvZnR3YXJlAF1w/zoAAAALdEVYdERpc2NsYWltZXIAt8C0jwAAAAh0RVh0V2FybmluZwDAG+aHAAAAB3RFWHRTb3VyY2UA9f+D6wAAAAh0RVh0Q29tbWVudAD2zJa/AAAABnRFWHRUaXRsZQCo7tInAAABV0lEQVQokaXSPWtTYRTA8d9N7k1zm6a+RG2x+FItgpu66uDQxbFurrr5OQQHR9FZnARB3PwSFqooddAStCBoqmLtS9omx+ESUXuDon94tnP+5+1JYm057GyQjZFP+l+S6G2FzlNe3WHtHc2TNI8zOlUUGLxsD1kDyR+EEQE2P/L8Jm/uk6RUc6oZaYM0JxtnpEX9AGPTtM6w7yzVEb61EaSNn4QD3j5m4QabH6hkVFLSUeqHyCeot0ib6BdNVGscPM/hWWr7S4Tw9TUvbpFUitHTnF6XrS+sL7O6VBSausT0FZonSkb+nZUFFm+z8Z5up5Btr1Lby7E5Zq4yPrMrLR263ZV52g+LvfW3iy6PXubUNVrnhqYNF3bmiZ1i1MmLnL7OxIWh4T+IMpYeRNyrRzyZjWg/ioh+aVgZu4WfXxaixbsRve5fiwb8epTo8+kZjSPFf/sHvgNC0/mbjJbxPAAAAABJRU5ErkJggg==";
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
let mut message = Message::new(
"mailjet_sender@company.com",
"Mailjet Rust",
Some("Your email flight plan!".to_string()),
Some("Dear passenger, welcome to Mailjet! May the delivery force be with you!".to_string())
);
message.set_receivers(
vec![
Recipient::new("bar@foo.com"),
],
Some(vec![
Recipient::new("bee@foo.com"),
]),
None
);
let mailjet_logo = Attachment::new(
"image/png",
"logo.png",
MAILJET_LOGO_BASE64);
message.attach_inline(mailjet_logo);
message.html_part = Some("<h3>Dear [[var:name]] [[var:last]], welcome to <img src=\"cid:logo.png\"> <a href=\"https://www.mailjet.com/\">Mailjet</a>!<br />May the delivery force be with you!".to_string());
let response = client.send(message).await;
println!("{:?}", response);
Ok(())
}
v3 上的功能齐全的示例
以下是一个使用 Mailjet 的 Send API v3 的示例,其中涵盖了以下功能
- 附加内联图像
- 附加文件
- 使用模板变量
use mailjet_rs::common::Recipient;
use mailjet_rs::v3::{Message, Attachment};
use mailjet_rs::{Client, SendAPIVersion};
use mailjet_rs::{Map, Value};
/// Base64 representation of the Mailjet logo found in the Mailjet SendAPI V3 docs
const MAILJET_LOGO_BASE64: &str = "iVBORw0KGgoAAAANSUhEUgAAABQAAAALCAYAAAB/Ca1DAAAACXBIWXMAAA7EAAAOxAGVKw4bAAAAB3RJTUUH4wIIChcxurq5eQAAAAd0RVh0QXV0aG9yAKmuzEgAAAAMdEVYdERlc2NyaXB0aW9uABMJISMAAAAKdEVYdENvcHlyaWdodACsD8w6AAAADnRFWHRDcmVhdGlvbiB0aW1lADX3DwkAAAAJdEVYdFNvZnR3YXJlAF1w/zoAAAALdEVYdERpc2NsYWltZXIAt8C0jwAAAAh0RVh0V2FybmluZwDAG+aHAAAAB3RFWHRTb3VyY2UA9f+D6wAAAAh0RVh0Q29tbWVudAD2zJa/AAAABnRFWHRUaXRsZQCo7tInAAABV0lEQVQokaXSPWtTYRTA8d9N7k1zm6a+RG2x+FItgpu66uDQxbFurrr5OQQHR9FZnARB3PwSFqooddAStCBoqmLtS9omx+ESUXuDon94tnP+5+1JYm057GyQjZFP+l+S6G2FzlNe3WHtHc2TNI8zOlUUGLxsD1kDyR+EEQE2P/L8Jm/uk6RUc6oZaYM0JxtnpEX9AGPTtM6w7yzVEb61EaSNn4QD3j5m4QabH6hkVFLSUeqHyCeot0ib6BdNVGscPM/hWWr7S4Tw9TUvbpFUitHTnF6XrS+sL7O6VBSausT0FZonSkb+nZUFFm+z8Z5up5Btr1Lby7E5Zq4yPrMrLR263ZV52g+LvfW3iy6PXubUNVrnhqYNF3bmiZ1i1MmLnL7OxIWh4T+IMpYeRNyrRzyZjWg/ioh+aVgZu4WfXxaixbsRve5fiwb8epTo8+kZjSPFf/sHvgNC0/mbjJbxPAAAAABJRU5ErkJggg==";
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
// Create an instance of the Mailjet API client
// used to send the `Message` and also define your API
// credentials
let client = Client::new(
SendAPIVersion::V3,
"public_key",
"private_key",
);
// Create your a `Message` instance with the minimum required values
let mut message = Message::new(
"mailjet_sender@company.com",
"Mailjet Rust",
Some("Your email flight plan!".to_string()),
Some("Dear passenger, welcome to Mailjet! May the delivery force be with you!".to_string())
);
message.push_recipient(Recipient::new("receiver@company.com"));
// Set some HTML for your email
//
// Note that here we are using `cid:logo.png` as the src value for our image
// this is using the `inline_attachment` with `filename` "logo.png" as the
// image source
message.html_part = Some("<h3>Dear [[var:name]] [[var:last]], welcome to <img src=\"cid:logo.png\"> <a href=\"https://www.mailjet.com/\">Mailjet</a>!<br />May the delivery force be with you!".to_string());
// Attach inline files providing its base64 representation
// content-type and a name.
// The name of the file can be used to reference this file in your HTML content
let mailjet_logo_inline = Attachment::new(
"image/png",
"logo.png",
MAILJET_LOGO_BASE64);
// Attach the `Attachment` as an Inline Attachment
// this function can also be used to attach common Attachments
message.attach_inline(mailjet_logo_inline);
// Creates a txt file Attachment
let txt_file_attachment = Attachment::new(
"text/plain",
"test.txt",
"VGhpcyBpcyB5b3VyIGF0dGFjaGVkIGZpbGUhISEK");
// Attaches the TXT file as an email Attachment
message.attach(txt_file_attachment);
// Provide variables for your template
// `Map` and `Value` are reexported from
// `serde_json`
let mut vars = Map::new();
vars.insert(String::from("name"), Value::from("Foo"));
vars.insert(String::from("last"), Value::from("Bar"));
message.vars = Some(vars);
// Finally send the message using the `Client`
let response = client.send(message).await;
// Do something with the response from Mailjet
// Ok(Response { sent: [Sent { email: "your_receiver@company.com", message_id: 000, message_uuid: "message-uuid" }] })
println!("{:?}", response);
Ok(())
}
发布
要发布新版本,您必须使用 git 标记并推送到 main
分支。
git tag -a v0.1.0 -m "First Release"
git push origin main --follow-tags
贡献
请随意贡献!
许可
该项目受 MIT 许可证的许可,与 Mailjet 的官方包装器具有相同的许可。
依赖关系
~7-22MB
~318K SLoC