9个版本
新 0.3.3 | 2024年8月21日 |
---|---|
0.3.2 | 2024年8月21日 |
0.2.1 | 2024年8月15日 |
0.1.3 |
|
0.1.2 | 2024年7月20日 |
#162 in 数学
842 每月下载量
65KB
567 行
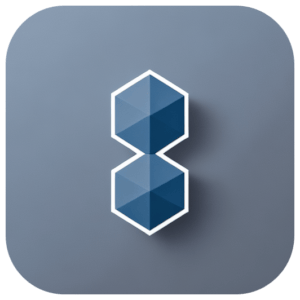
Hexing
"六边形是最好的六边形"
一个基本的Rust库,用于操作六边形网格。
hexing
是一个Rust库,旨在处理和计算六边形网格。它提供用于处理六边形位置和方向的工具,以及用于探索六边形环和螺旋的迭代器。
功能
- 六边形坐标操作:使用轴坐标表示和操作六边形网格中的位置。
- 距离计算:计算两个六边形位置之间的距离。
- 像素坐标转换:将六边形位置转换为像素坐标以供图形使用。
- 反射和旋转:将反射和旋转应用于六边形位置。
- 环和螺旋迭代器:获取围绕中心位置环或螺旋中的位置。
- 边界:计算一个点是否在六边形边界框内。
- 线迭代器:获取两个六边形位置之间的线上的位置。
- 数字特质:允许使用各种数值类型进行泛型计算。
- 序列化和反序列化:使用
serde
功能序列化和反序列化六边形位置和方向。 - 路径查找:使用A*算法在两个六边形位置之间找到最短路径。
- 视野:计算六边形网格的视野。
- 行动场:计算六边形网格的行动场。
- 噪声生成:使用
noise
crate为六边形网格生成噪声图。
关于Serde功能的文档位于docs/serde.md文件中。路径查找、视野、行动场和噪声生成功能的文档位于docs/layout.md文件中。
数字特质
该库使用Number
特质来允许使用各种数值类型进行泛型计算。此特质为几种类型实现,包括整数和浮点数。
主要类型
HexPosition<T>
表示具有坐标T
的六边形网格中的位置。坐标为轴格式(x, y)
。
-
创建:使用给定的
q
和r
坐标在轴向格式中创建一个新的 [HexPosition]。坐标解释见此 文档。let pos = HexPosition::new(1, 2); let pos2 = HexPosition(3, 4); // Constant: The origin of the hexagonal grid. let origin = HexPosition::ORIGIN;
-
转换为像素坐标:将当前 [HexPosition] 转换为像素坐标。基本上,它将六边形网格中的一个位置转换为正交网格中的一个位置。
let pixel_coords = pos.to_pixel_coordinates(); let pixel_coords2 = HexPosition::from_pixel_coordinates(pixel_coords);
-
距离计算:使用 曼哈顿距离 计算两个六边形位置之间的距离。
let distance = pos.distance(HexPosition::new(3, 4));
-
旋转:将对原点进行 2 x 60 度的旋转。
let rotated_pos = pos.rotation(2);
-
反射:将在原点周围进行中心对称反射。
let reflected_pos = pos.reflect();
-
环迭代器:一个迭代器,返回围绕中心位置的环上的位置。迭代器将返回给定半径的环上的位置。
for ring_pos in pos.ring(2) { println!("{:?}", ring_pos); }
-
螺旋迭代器:一个迭代器,返回围绕中心位置的螺旋上的位置。迭代器将返回给定半径的螺旋上的位置。
for spiral_pos in pos.spiral(2) { println!("{:?}", spiral_pos); }
-
线迭代器:一个迭代器,返回两个六边形位置之间的线上的位置。
let a = HexPosition(0, 0); let b = HexPosition(-2, -1); for line_pos in a.line(b) { println!("{:?}", line_pos); }
-
边界:一个实用函数,用于计算六边形网格的边界框。
use hexing::{utils::HexBound, HexPosition}; let center = HexPosition(0, 0); let radius = 3; let bounding_box = HexBound::new(center, radius); assert!(bounding_box.contains(HexPosition(0, 0))); assert_eq!(bounding_box.radius(), 3); assert_eq!(bounding_box.center(), HexPosition(0, 0));
HexDirection
枚举,表示六边形网格中的六个可能方向。
-
可用方向:
右
(1, 0)右上
(1, -1)左上
(0, -1)左
(-1, 0)左下
(-1, 1)右下
(0, 1)
-
转换为向量:您可以使用
to_vector
方法将 [HexDirection] 转换为 [HexPosition]。let direction = HexDirection::Right; let vector = direction.to_vector();
用法示例
以下是一些示例,说明 hexing
的功能。
创建六边形位置
use hexing::HexPosition;
let pos = HexPosition::new(1, 2);
let pos2 = HexPosition(3, 4);
let origin = HexPosition::ORIGIN;
println!("Position 1: {:?}", pos);
println!("Position 2: {:?}", pos2);
println!("Origin: {:?}", origin);
转换为像素坐标
use hexing::HexPosition;
let position = HexPosition::new(1, 0);
let pixel_coords = position.to_pixel_coordinates();
println!("Pixel coordinates: {:?}", pixel_coords);
let new_position: HexPosition<i32> = HexPosition::from_pixel_coordinates(pixel_coords);
println!("New position: {:?}", new_position);
assert!(position == new_position);
计算位置之间的距离
use hexing::HexPosition;
let pos1 = HexPosition::new(0, 0);
let pos2 = HexPosition::new(-2, -1);
let dist = pos1.distance(pos2);
println!("Distance: {:?}", dist);
遍历环和螺旋
use hexing::{HexPosition, HexRing, HexSpiral};
let center = HexPosition::new(0, 0);
// Ring of radius 1
let ring = center.ring(1);
for pos in ring {
println!("Ring position: {:?}", pos);
}
// Spiral of radius 2
let spiral = center.spiral(2);
for pos in spiral {
println!("Spiral position: {:?}", pos);
}
旋转六边形位置
use hexing::HexPosition;
let rotation = 120;
let pos = HexPosition::new(1, 2);
let rotated_pos = pos.rotation(rotation/60); // Rotates 120 degrees
println!("Rotated Position: {:?}", rotated_pos);
反射六边形位置
use hexing::HexPosition;
let pos = HexPosition::new(1, 2);
let reflected_pos = pos.reflect();
println!("Reflected Position: {:?}", reflected_pos);
线迭代器
use hexing::HexPosition;
let start = HexPosition::new(0, 0);
let end = HexPosition::new(3, -3);
for pos in start.line(end) {
println!("Line Position: {:?}", pos);
}
使用 HexDirection
use hexing::HexDirection;
let direction = HexDirection::UpRight;
let vector = direction.to_vector();
println!("Vector for Right Direction: {:?}", vector);
let new_position = HexPosition::new(0, 0) + vector * 3;
println!("New Position after moving Right: {:?}", new_position);
完整文档
有关六边形网格的更多详细文档和解释,请参阅 Red Blob Games 六边形网格文档。
安装
将 hexing
添加到您的 Cargo.toml
[dependencies]
hexing = "0.3.3"
依赖项
~0.8–1.3MB
~23K SLoC