109 个版本
0.25.2 | 2024 年 7 月 21 日 |
---|---|
0.25.1 | 2024 年 3 月 31 日 |
0.25.0-preview.0 | 2024 年 2 月 25 日 |
0.24.7 | 2023 年 8 月 9 日 |
0.2.0-alpha.2 | 2014 年 11 月 22 日 |
在 图像 中排名 13
每月下载量 1,976,266
在 4,730 个crate中使用(直接使用2,447个)
1MB
20K SLoC
Image
维护者: @HeroicKatora、@fintelia
图像处理库
此crate提供基本的图像处理函数以及将图像格式相互转换的方法。
提供的所有图像处理函数都操作实现GenericImageView
和GenericImage
特质的类型,并返回一个ImageBuffer
。
高级API
使用ImageReader
加载图像
use std::io::Cursor;
use image::ImageReader;
let img = ImageReader::open("myimage.png")?.decode()?;
let img2 = ImageReader::new(Cursor::new(bytes)).with_guessed_format()?.decode()?;
使用save
或write_to
方法保存它们
img.save("empty.jpg")?;
let mut bytes: Vec<u8> = Vec::new();
img2.write_to(&mut Cursor::new(&mut bytes), image::ImageFormat::Png)?;
支持的图像格式
默认功能启用时,image
提供了许多常见图像格式编解码器的实现。
格式 | 解码 | 编码 |
---|---|---|
AVIF | 是(仅限8位) * | 是(仅限有损) |
BMP | 是 | 是 |
DDS | 是 | --- |
Farbfeld | 是 | 是 |
GIF | 是 | 是 |
HDR | 是 | 是 |
ICO | 是 | 是 |
JPEG | 是 | 是 |
EXR | 是 | 是 |
PNG | 是 | 是 |
PNM | 是 | 是 |
QOI | 是 | 是 |
TGA | 是 | 是 |
TIFF | 是 | 是 |
WebP | 是 | 是(仅限无损) |
- * 需要启用
avif-native
功能,使用libdav1d C库。
图像类型
此软件包提供多种不同类型来表示图像。图像中的单个像素以(0,0)坐标从左上角开始索引。
ImageBuffer
ImageBuffer 是一个通过像素类型、宽度和高度以及像素向量来参数化的图像。它提供对其像素的直接访问,并实现了 GenericImageView
和 GenericImage
特性。
DynamicImage
DynamicImage 是一个枚举,涵盖所有支持的 ImageBuffer<P>
类型。其确切图像类型在运行时确定。打开图像时返回此类型。为了方便,DynamicImage
重新实现了所有图像处理函数。
GenericImageView
和 GenericImage
特性
这些特性提供了用于检查(GenericImageView
)和操作(GenericImage
)图像的方法,这些方法参数化图像的像素类型。
SubImage
SubImage 是另一个图像的视图,由矩形坐标界定。给定的坐标设置矩形左上角的位置。这用于在图像的子区域内执行图像处理函数。
ImageDecoder
和 ImageDecoderRect
特性
所有图像格式解码器都实现了 ImageDecoder
特性,它提供了获取图像元数据和解码图像的基本方法。某些格式还提供了 ImageDecoderRect
实现,允许一次性解码图像的一部分。
解码器最重要的方法是...
- dimensions:返回包含图像宽度和高度的元组。
- color_type:返回由此解码器产生的图像数据的颜色类型。
- read_image:将整个图像解码到字节数组切片中。
像素
image
提供以下像素类型
- Rgb:RGB 像素
- Rgba:带有 alpha 的 RGB(RGBA 像素)
- Luma:灰度像素
- LumaA:带有 alpha 的灰度
所有像素都是通过其组件类型参数化的。
图像处理函数
这些是在 imageops
模块中定义的函数。所有函数都作用于实现了 GenericImage
特性的类型。请注意,某些函数在调试模式下非常慢。如果遇到任何性能问题,请确保使用发布模式。
- blur:对提供的图像执行高斯模糊。
- brighten:使提供的图像变亮。
- huerotate:按度数旋转提供的图像。
- contrast:调整提供的图像的对比度。
- crop:返回对图像的不可变视图。
- filter3x3:对提供的图像执行 3x3 矩形滤波。
- flip_horizontal:水平翻转图像。
- flip_vertical:垂直翻转图像。
- grayscale:将提供的图像转换为灰度。
- invert:反转提供的图像中的每个像素。此函数就地操作。
- resize:将提供的图像调整到指定的尺寸。
- rotate180:顺时针旋转图像 180 度。
- rotate270:顺时针旋转图像 270 度。
- rotate90:顺时针旋转图像 90 度。
- unsharpen:对提供的图像执行非锐化掩码。
有关更多选项,请参阅 imageproc
crate。
示例
打开和保存图像
image
提供了用于从路径打开图像的 open
函数。图像格式由路径的文件扩展名确定。一个 io
模块提供了一个提供更多控制的读取器。
use image::GenericImageView;
// Use the open function to load an image from a Path.
// `open` returns a `DynamicImage` on success.
let img = image::open("tests/images/jpg/progressive/cat.jpg").unwrap();
// The dimensions method returns the images width and height.
println!("dimensions {:?}", img.dimensions());
// The color method returns the image's `ColorType`.
println!("{:?}", img.color());
// Write the contents of this image to the Writer in PNG format.
img.save("test.png").unwrap();
生成分形
//! An example of generating julia fractals.
let imgx = 800;
let imgy = 800;
let scalex = 3.0 / imgx as f32;
let scaley = 3.0 / imgy as f32;
// Create a new ImgBuf with width: imgx and height: imgy
let mut imgbuf = image::ImageBuffer::new(imgx, imgy);
// Iterate over the coordinates and pixels of the image
for (x, y, pixel) in imgbuf.enumerate_pixels_mut() {
let r = (0.3 * x as f32) as u8;
let b = (0.3 * y as f32) as u8;
*pixel = image::Rgb([r, 0, b]);
}
// A redundant loop to demonstrate reading image data
for x in 0..imgx {
for y in 0..imgy {
let cx = y as f32 * scalex - 1.5;
let cy = x as f32 * scaley - 1.5;
let c = num_complex::Complex::new(-0.4, 0.6);
let mut z = num_complex::Complex::new(cx, cy);
let mut i = 0;
while i < 255 && z.norm() <= 2.0 {
z = z * z + c;
i += 1;
}
let pixel = imgbuf.get_pixel_mut(x, y);
let image::Rgb(data) = *pixel;
*pixel = image::Rgb([data[0], i as u8, data[2]]);
}
}
// Save the image as “fractal.png”, the format is deduced from the path
imgbuf.save("fractal.png").unwrap();
示例输出
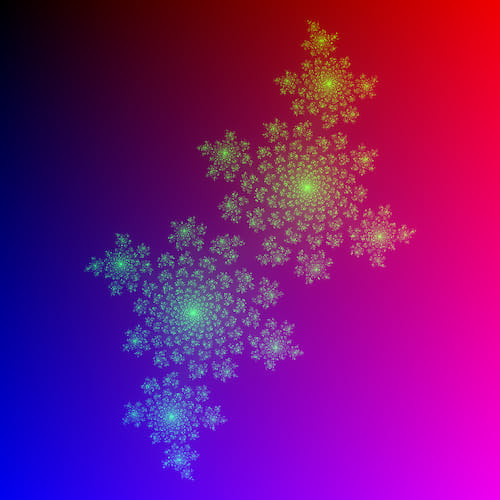
写入原始缓冲区
如果不需要高级接口,因为图像是通过其他方式获得的,image
提供了将缓冲区保存到文件的函数 save_buffer
。
let buffer: &[u8] = unimplemented!(); // Generate the image data
// Save the buffer as "image.png"
image::save_buffer("image.png", buffer, 800, 600, image::ExtendedColorType::Rgb8).unwrap()
依赖关系
~1.5–6MB
~114K SLoC