10个版本 (稳定版)
2.1.0 | 2024年1月14日 |
---|---|
2.0.1 | 2022年2月15日 |
1.1.2 | 2022年1月20日 |
0.2.0 | 2022年1月14日 |
0.1.1 | 2022年1月14日 |
#633 在 测试
每月62次 下载
在 mutils 中使用
310KB
422 行
TestCat
TestCat是一组宏,使您能够以更可读的方式维护测试。通过允许将测试用例在文件顶部组合在一起。
它基于JavaScript测试库Jest。
宏包括...
it
和test
describe
目标
目标是提高可读性。使管理包含大量测试的长文件变得更简单。
通过将测试用例分组在一起。它可以让您一眼看出有哪些测试用例。这对于代码审查特别有用。
完整示例
简而言之,它允许您像这样记录测试用例...
#[cfg(test)]
mod angle_to {
use super::*;
use ::testcat::*;
use ::assert_approx_eq::assert_approx_eq;
use ::std::f32::consts::TAU;
describe!("angle to zero", {
it!("should angle to zero from right", test_angle_to_zero_from_right);
it!("should angle to zero from above", test_angle_to_zero_from_above);
it!("should angle to zero from left", test_angle_to_zero_from_left);
it!("should angle to zero from below", test_angle_to_zero_from_below);
});
describe!("angle to point", {
it!("should angle to point from right", test_angle_to_point_from_right);
it!("should angle to point from above", test_angle_to_point_from_above);
it!("should angle to point from left", test_angle_to_point_from_left);
it!("should angle to point from below", test_angle_to_point_from_below);
});
fn test_angle_to_zero_from_right() {
// code omitted
}
// rest of test functions omitted
运行 cargo test
,您将得到以下输出...
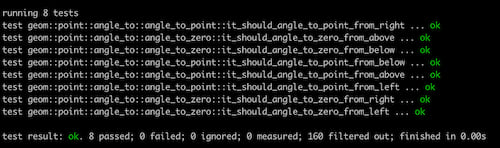
it
和 test
宏
it
和 test
是相同的宏,允许您在顶部一起列出测试用例。这些宏转换为一个包装函数,该函数调用您的测试。宏接受测试的描述和该测试的函数名称。
有两种版本可帮助提高可读性。它们的行为相同。
- 当说明期望的结果时应该使用
it
。例如,it!("should give the user a cookie", test_give_cookie)
。 - 对于其他类型的更通用的测试应该使用
test
。例如,test!("cookie dispenser runs", test_cookie_dispenser)
。
示例代码
#[cfg(test)]
mod testing {
use ::testcat::*;
it!("should allow the user to do x", test_user_does_x);
it!("should not allow the user to do y", test_y_disallowed);
test!("foobobulator doesn't crash", test_foobobulator);
fn test_user_does_x() {
// code omitted
}
fn test_y_disallowed() {
// code omitted
}
fn test_foobobulator() {
// code omitted
}
}
模块名称
您还可以使用模块路径作为测试名称。
#[cfg(test)]
mod testing {
use ::testcat::*;
it!("should allow the user to do x", test_use::test_does_x);
it!("should not allow the user to do y", test_use::test_y_disallowed);
test!("foobobulator doesn't crash", test_foo::test_foobobulator);
mod test_use {
pub fn test_does_x() {
// code omitted
}
pub fn test_y_disallowed() {
// code omitted
}
}
mod test_foo {
pub fn test_foobobulator() {
// code omitted
}
}
}
describe
宏
describe
块用于将相似的测试分组在一起。这些块将转换为一个子模块,其中列出测试。
示例代码
#[cfg(test)]
mod testing {
use ::testcat::*;
describe("user interaction", {
it!("should allow the user to do x", test_user_does_x);
it!("should not allow the user to do y", test_y_disallowed);
})
describe("timing", {
it!("should do foo before bar", test_foo_over_bar);
})
fn test_user_does_x() {
// code omitted
}
fn test_y_disallowed() {
// code omitted
}
fn test_foo_over_bar() {
// code omitted
}
}
示例 2
describe
块也可以包含函数。因为这是一个子模块的代码。
#[cfg(test)]
mod testing {
use ::testcat::*;
describe("user interaction", {
it!("should allow the user to do x", test_user_does_x);
it!("should not allow the user to do y", test_y_disallowed);
fn test_user_does_x() {
// code omitted
}
fn test_y_disallowed() {
// code omitted
}
})
describe("timing", {
it!("should do foo before bar", test_foo_over_bar);
fn test_foo_over_bar() {
// code omitted
}
})
}