16个版本
0.8.0 | 2024年8月15日 |
---|---|
0.7.1 | 2023年1月17日 |
0.7.0 | 2022年10月6日 |
0.6.3 | 2022年7月19日 |
0.2.1 | 2020年2月24日 |
996 在 过程宏 中
9,909 每月下载量
在 33 个包中使用(直接使用3个)
42KB
951 行
亮点
-
声明式设计。
teloxide
基于dptree
,这是一种功能性的 责任链模式,它允许你以高度声明性和可扩展的方式表达消息处理管道。 -
功能丰富。 你可以使用长轮询和webhooks,配置底层的HTTPS客户端,设置自定义的Telegram API服务器URL,优雅地关闭,等等。
-
简单的对话。 我们的对话子系统简单易用,并且对对话的存储方式/位置无关。例如,你只需替换一行即可实现 持久化。开箱即用的存储包括 Redis 和 Sqlite。
-
强类型命令。 将机器人命令定义为
enum
,teloxide 将自动解析它们——就像serde-json
中的JSON结构体以及在structopt
中的命令行参数。
设置你的环境
- 下载Rust.
- 使用 @Botfather 创建一个新机器人以获取格式为
123456789:blablabla
的令牌。 - 将环境变量
TELOXIDE_TOKEN
初始化为您的令牌
# Unix-like
$ export TELOXIDE_TOKEN=<Your token here>
# Windows command line
$ set TELOXIDE_TOKEN=<Your token here>
# Windows PowerShell
$ $env:TELOXIDE_TOKEN=<Your token here>
- 确保您的 Rust 编译器是最新的(目前
teloxide
需要 rustc 至少版本 1.70)
# If you're using stable
$ rustup update stable
$ rustup override set stable
# If you're using nightly
$ rustup update nightly
$ rustup override set nightly
- 运行
cargo new my_bot
,进入目录并在您的Cargo.toml
中添加以下行
[dependencies]
teloxide = { version = "0.13", features = ["macros"] }
log = "0.4"
pretty_env_logger = "0.4"
tokio = { version = "1.8", features = ["rt-multi-thread", "macros"] }
API 概览
骰子机器人
该机器人会对收到的每条消息回复一个骰子
use teloxide::prelude::*;
#[tokio::main]
async fn main() {
pretty_env_logger::init();
log::info!("Starting throw dice bot...");
let bot = Bot::from_env();
teloxide::repl(bot, |bot: Bot, msg: Message| async move {
bot.send_dice(msg.chat.id).await?;
Ok(())
})
.await;
}
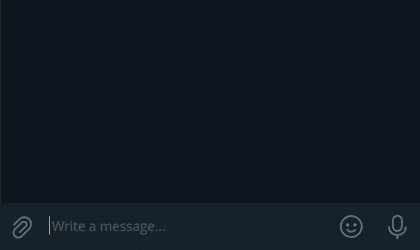
命令
命令是强类型且声明性的,类似于我们使用 structopt 定义 CLI 和在 serde-json 中定义 JSON 结构。以下机器人接受这些命令
/用户名<您的用户名>
/usernameandage<您的用户名> <您的年龄>
/help
use teloxide::{prelude::*, utils::command::BotCommands};
#[tokio::main]
async fn main() {
pretty_env_logger::init();
log::info!("Starting command bot...");
let bot = Bot::from_env();
Command::repl(bot, answer).await;
}
#[derive(BotCommands, Clone)]
#[command(rename_rule = "lowercase", description = "These commands are supported:")]
enum Command {
#[command(description = "display this text.")]
Help,
#[command(description = "handle a username.")]
Username(String),
#[command(description = "handle a username and an age.", parse_with = "split")]
UsernameAndAge { username: String, age: u8 },
}
async fn answer(bot: Bot, msg: Message, cmd: Command) -> ResponseResult<()> {
match cmd {
Command::Help => bot.send_message(msg.chat.id, Command::descriptions().to_string()).await?,
Command::Username(username) => {
bot.send_message(msg.chat.id, format!("Your username is @{username}.")).await?
}
Command::UsernameAndAge { username, age } => {
bot.send_message(msg.chat.id, format!("Your username is @{username} and age is {age}."))
.await?
}
};
Ok(())
}
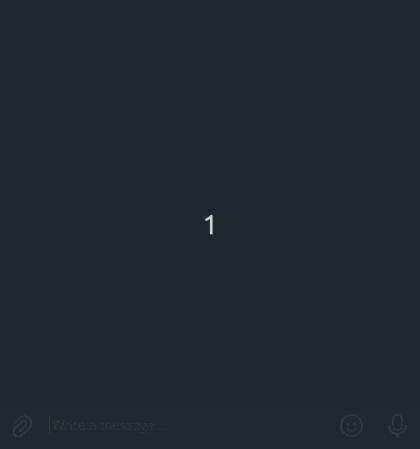
对话管理
对话通常由一个枚举来描述,每个变体代表对话的一种可能状态。还有 状态处理函数,它们可以将对话从一个状态转换为另一个状态,从而形成一个 有限状态机。
以下是一个机器人,它会问您三个问题,然后把答案发回给您
use teloxide::{dispatching::dialogue::InMemStorage, prelude::*};
type MyDialogue = Dialogue<State, InMemStorage<State>>;
type HandlerResult = Result<(), Box<dyn std::error::Error + Send + Sync>>;
#[derive(Clone, Default)]
pub enum State {
#[default]
Start,
ReceiveFullName,
ReceiveAge {
full_name: String,
},
ReceiveLocation {
full_name: String,
age: u8,
},
}
#[tokio::main]
async fn main() {
pretty_env_logger::init();
log::info!("Starting dialogue bot...");
let bot = Bot::from_env();
Dispatcher::builder(
bot,
Update::filter_message()
.enter_dialogue::<Message, InMemStorage<State>, State>()
.branch(dptree::case![State::Start].endpoint(start))
.branch(dptree::case![State::ReceiveFullName].endpoint(receive_full_name))
.branch(dptree::case![State::ReceiveAge { full_name }].endpoint(receive_age))
.branch(
dptree::case![State::ReceiveLocation { full_name, age }].endpoint(receive_location),
),
)
.dependencies(dptree::deps![InMemStorage::<State>::new()])
.enable_ctrlc_handler()
.build()
.dispatch()
.await;
}
async fn start(bot: Bot, dialogue: MyDialogue, msg: Message) -> HandlerResult {
bot.send_message(msg.chat.id, "Let's start! What's your full name?").await?;
dialogue.update(State::ReceiveFullName).await?;
Ok(())
}
async fn receive_full_name(bot: Bot, dialogue: MyDialogue, msg: Message) -> HandlerResult {
match msg.text() {
Some(text) => {
bot.send_message(msg.chat.id, "How old are you?").await?;
dialogue.update(State::ReceiveAge { full_name: text.into() }).await?;
}
None => {
bot.send_message(msg.chat.id, "Send me plain text.").await?;
}
}
Ok(())
}
async fn receive_age(
bot: Bot,
dialogue: MyDialogue,
full_name: String, // Available from `State::ReceiveAge`.
msg: Message,
) -> HandlerResult {
match msg.text().map(|text| text.parse::<u8>()) {
Some(Ok(age)) => {
bot.send_message(msg.chat.id, "What's your location?").await?;
dialogue.update(State::ReceiveLocation { full_name, age }).await?;
}
_ => {
bot.send_message(msg.chat.id, "Send me a number.").await?;
}
}
Ok(())
}
async fn receive_location(
bot: Bot,
dialogue: MyDialogue,
(full_name, age): (String, u8), // Available from `State::ReceiveLocation`.
msg: Message,
) -> HandlerResult {
match msg.text() {
Some(location) => {
let report = format!("Full name: {full_name}\nAge: {age}\nLocation: {location}");
bot.send_message(msg.chat.id, report).await?;
dialogue.exit().await?;
}
None => {
bot.send_message(msg.chat.id, "Send me plain text.").await?;
}
}
Ok(())
}
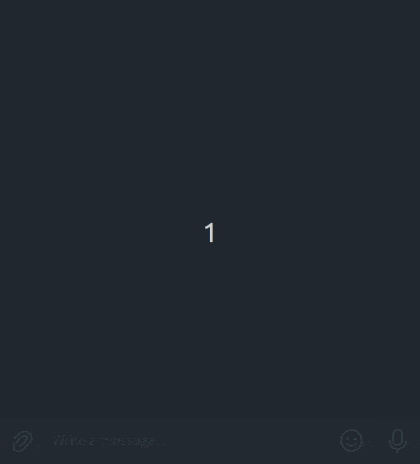
测试
一个社区制作的 teloxide_tests
可以用来测试您的机器人。
教程
- "Migrating my family finance bot from Python to Rust (teloxide) because I am tired of exceptions (part 1)" by Troy Köhler.
- "Migrating my family finance bot from Python to Rust (teloxide) [part 2]" by Troy Köhler.
常见问题解答
问题:我可以在哪里提问?
A
- 问题 是关于库设计、增强和错误报告的良好提问场所。
- GitHub 讨论区 是一个可以以不那么正式的方式向我们寻求帮助的地方。
- 如果您需要实时快速的帮助,请在 我们的官方 Telegram 群组 中提问。
问题:您支持客户端的 Telegram API 吗?
A: 不,只支持机器人 API。
问题:我可以使用 webhooks 吗?
A: 可以!teloxide
在 dispatching::update_listeners::webhooks
模块中内置了对 webhooks 的支持。请参考 examples/ngrok_ping_pong_bot
和 examples/heroku_ping_pong_bot
中的使用方法。
问题:我能否在单个对话中处理回调查询和消息?
A: 是的,请参考 examples/purchase.rs
。
社区机器人
欢迎您提出自己的机器人加入我们的收藏!
raine/tgreddit
— 一个将你最喜欢的subreddits的顶级帖子发送到Telegram的机器人。magnickolas/remindee-bot
— 用于管理提醒的Telegram机器人。WaffleLapkin/crate_upd_bot
— 通知crate更新的机器人。mattrighetti/GroupActivityBot
— 跟踪组内用户活动的Telegram机器人。alenpaul2001/AurSearchBot
— 在Arch User Repository (AUR)中进行搜索的Telegram机器人。ArtHome12/vzmuinebot
— 用于食物菜单导航的Telegram机器人。studiedlist/EddieBot
— 具有多个娱乐功能的聊天机器人。modos189/tg_blackbox_bot
— 你的Telegram项目的匿名反馈。0xNima/spacecraft
— 另一个用于下载Twitter空间的Telegram机器人。0xNima/Twideo
— 简单的Telegram机器人,用于通过链接从Twitter下载视频。mattrighetti/libgen-bot-rs
— 与libgen接口的Telegram机器人。zamazan4ik/npaperbot-telegram
— 通过C++提案进行搜索的Telegram机器人。studentenherz/dlebot
— 一个查询西班牙语词典中单词定义的机器人。fr0staman/fr0staman_bot
— 具有丰富功能的Telegram游戏类机器人,附带猪🐷。franciscofigueira/transferBot
— 通知加密代币转账的Telegram机器人。
显示使用`teloxide`且版本低于v0.6.0的机器人
mxseev/logram
— 从任何地方获取日志并发送到Telegram的实用程序。alexkonovalov/PedigreeBot
— 用于构建家谱的Telegram机器人。Hermitter/tepe
— 用于通过Telegram发送消息和文件的命令行界面。myblackbeard/basketball-betting-bot
— 机器人让你可以对NBA比赛进行赌注,与你的朋友对抗。dracarys18/grpmr-rs
— 使用Rust编写的模块化Telegram群组管理机器人。ArtHome12/cognito_bot
— 该机器人的设计旨在匿名化群组中的消息。crapstone/hsctt
— 一个将HTTP状态码转换为文本的机器人。
查看使用 teloxide
的 1900+ 个其他公开仓库 >>
贡献
查看 CONRIBUTING.md
。
依赖
~1.5MB
~36K SLoC