73个重大版本
新 0.74.0 | Aug 23, 2024 |
---|---|
0.72.0 | Jul 26, 2024 |
0.64.1 | Mar 26, 2024 |
0.59.0 | Dec 15, 2023 |
0.1.0 | Jun 17, 2022 |
#51 in 编码
22,070 downloads per month
Used in 17 crates (16 directly)
3.5MB
37K SLoC
Contains (Cab file, 18KB) examples/vbaProject.bin, (Cab file, 14KB) tests/input/macros/vbaProject01.bin, (Cab file, 13KB) tests/input/macros/vbaProject02.bin, (Cab file, 14KB) tests/input/macros/vbaProject03.bin, (Cab file, 14KB) tests/input/macros/vbaProject04.bin, (Cab file, 16KB) tests/input/macros/vbaProject05.bin
rust_xlsxwriter
The rust_xlsxwriter
library is a Rust library for writing Excel files in the xlsx format.
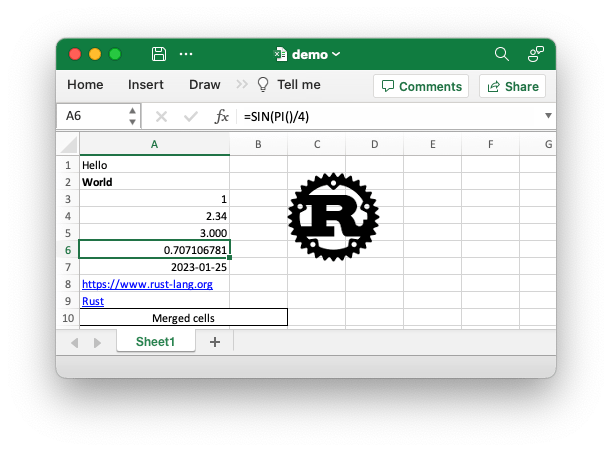
The rust_xlsxwriter
library can be used to write text, numbers, dates and formulas to multiple worksheets in a new Excel 2007+ xlsx file. It has a focus on performance and on fidelity with the file format created by Excel. It cannot be used to modify an existing file.
示例
生成上述Excel文件的示例代码。
use rust_xlsxwriter::*;
fn main() -> Result<(), XlsxError> {
// Create a new Excel file object.
let mut workbook = Workbook::new();
// Create some formats to use in the worksheet.
let bold_format = Format::new().set_bold();
let decimal_format = Format::new().set_num_format("0.000");
let date_format = Format::new().set_num_format("yyyy-mm-dd");
let merge_format = Format::new()
.set_border(FormatBorder::Thin)
.set_align(FormatAlign::Center);
// Add a worksheet to the workbook.
let worksheet = workbook.add_worksheet();
// Set the column width for clarity.
worksheet.set_column_width(0, 22)?;
// Write a string without formatting.
worksheet.write(0, 0, "Hello")?;
// Write a string with the bold format defined above.
worksheet.write_with_format(1, 0, "World", &bold_format)?;
// Write some numbers.
worksheet.write(2, 0, 1)?;
worksheet.write(3, 0, 2.34)?;
// Write a number with formatting.
worksheet.write_with_format(4, 0, 3.00, &decimal_format)?;
// Write a formula.
worksheet.write(5, 0, Formula::new("=SIN(PI()/4)"))?;
// Write a date.
let date = ExcelDateTime::from_ymd(2023, 1, 25)?;
worksheet.write_with_format(6, 0, &date, &date_format)?;
// Write some links.
worksheet.write(7, 0, Url::new("https://rust-lang.net.cn"))?;
worksheet.write(8, 0, Url::new("https://rust-lang.net.cn").set_text("Rust"))?;
// Write some merged cells.
worksheet.merge_range(9, 0, 9, 1, "Merged cells", &merge_format)?;
// Insert an image.
let image = Image::new("examples/rust_logo.png")?;
worksheet.insert_image(1, 2, &image)?;
// Save the file to disk.
workbook.save("demo.xlsx")?;
Ok(())
}
rust_xlsxwriter
is a rewrite of the Python XlsxWriter
library in Rust by the same author and with some additional Rust-like features and APIs. The currently supported features are
- 支持写入所有基本Excel数据类型。
- 完整的单元格格式化支持。
- 公式支持,包括新的Excel 365动态函数。
- 图表。
- 超链接支持。
- 页面/打印设置支持。
- 合并范围。
- 条件格式化。
- 数据验证。
- 单元格注释。
- Sparklines。
- 工作表PNG/JPEG/GIF/BMP图片。
- 富多格式字符串。
- 定义名称。
- 自动筛选。
- 工作表表格。
- Serde序列化支持。
- 支持宏。
rust_xlsxwriter
is under active development and new features will be added frequently.
特性
-
default
: Includes all the standard functionality. Has dependencies onzip
andregex
only. -
serde
:增加了对Serde序列化的支持。默认情况下是关闭的。 -
chrono
:为API增加了对Chrono日期/时间类型的支持。默认情况下是关闭的。 -
zlib
:添加了对zlib
和C编译器的依赖。这包括与default
相同的特性,但对于大文件速度可提高1.5倍。 -
polars
:添加了对PolarsError
和rust_xlsxwriter::XlsxError
之间映射的支持,以便于编写处理这两种类型错误的代码。 -
wasm
:添加了对js-sys
和wasm-bindgen
的依赖,以便允许为wasm/JavaScript目标编译。 -
ryu
:添加了对ryu
的依赖。这提高了为大数据文件编写数字工作表单元格的速度。当数字单元格超过300,000个时,性能将得到提升,并且比默认的数字格式快30%,对于5,000,000个数字单元格,速度可以快30%。
发行说明
最近的变化
- 增加了添加单元格注释的功能。
- 增加了添加VBA宏的功能。
- 增加了数据验证的功能。
查看完整的发行说明和变更日志。
另请参阅
- 用户指南:使用
rust_xlsxwriter
库。 - rust_xlsxwriter包.
- docs.rs上的rust_xlsxwriter API文档.
- rust_xlsxwriter仓库.
- 计划功能的路线图.
依赖关系
~4–18MB
~200K SLoC