2个稳定版本
新增 1.4.0 | 2024年8月17日 |
---|---|
1.3.0 | 2024年2月8日 |
#1414 in 网络编程
77 每月下载量
165KB
3.5K SLoC
netcode
rust实现
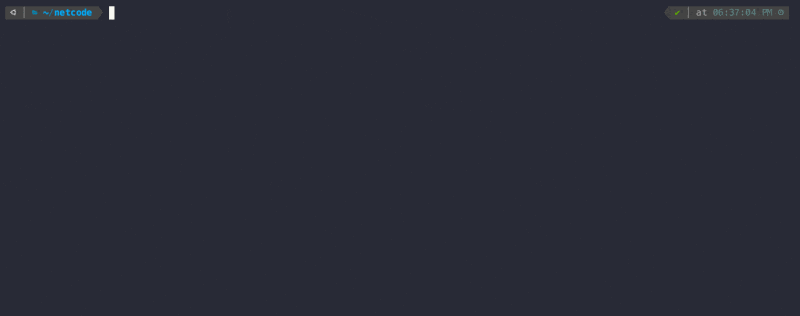
协议
此crate实现了由 Glenn Fiedler 创建的 netcode 网络协议。
netcode
是一个基于UDP的简单连接客户端/服务器协议。
请参阅官方源 规范 了解协议的工作方式。
安装
在您的项目目录中运行以下Cargo命令
cargo add netcode-rs
或者将以下行添加到您的Cargo.toml中
[dependencies]
netcode-rs = "1.4.0"
注意:虽然crate名称为netcode-rs
,但库的实际名称是netcode
。
这意味着,尽管您使用了cargo add netcode-rs
来添加依赖项,但您需要编写use netcode::*
(而不是use netcode_rs::*
)来使用它。
我知道这很令人困惑,我知道-rs
crate名称是不受欢迎的。
不幸的是,原始的netcode
crate不再维护,其维护者无法联系,因此我无法获得crate名称的所有权。我不想使用像netcode2
这样的名称,以免与netcode协议版本混淆。
示例
服务器
use std::{thread, time::{Instant, Duration}};
use netcode::{Server, MAX_PACKET_SIZE};
// Create a server
let protocol_id = 0x11223344u64; // a unique number that must match the client's protocol id
let private_key = netcode::generate_key(); // you can also provide your own key
let mut server = Server::new("0.0.0.0:5555", protocol_id, private_key).unwrap();
// Run the server at 60Hz
let start = Instant::now();
let tick_rate = Duration::from_secs_f64(1.0 / 60.0);
loop {
let elapsed = start.elapsed().as_secs_f64();
server.update(elapsed);
while let Some((packet, from)) = server.recv() {
// ...
}
thread::sleep(tick_rate);
}
客户端
use std::{thread, time::{Instant, Duration}};
use netcode::{ConnectToken, Client, MAX_PACKET_SIZE};
// Generate a connection token for the client
let protocol_id = 0x11223344u64; // a unique number that must match the server's protocol id
let private_key = netcode::generate_key(); // you can also provide your own key
let client_id = 123u64; // globally unique identifier for an authenticated client
let server_address = "my-domain.com:5555"; // the server's public address (can also be multiple addresses)
let connect_token = ConnectToken::build(server_address, protocol_id, client_id, private_key).generate().unwrap();
// Start the client
let token_bytes = connect_token.try_into_bytes().unwrap();
let mut client = Client::new(&token_bytes).unwrap();
client.connect();
// Run the client at 60Hz
let start = Instant::now();
let tick_rate = Duration::from_secs_f64(1.0 / 60.0);
loop {
let elapsed = start.elapsed().as_secs_f64();
client.update(elapsed);
if let Some(packet) = client.recv() {
// ...
}
thread::sleep(tick_rate);
}
更多示例请参阅 示例。
计划中的功能
依赖项
~1.5–9MB
~80K SLoC