2 个稳定版本
3.5.3 | 2024年6月10日 |
---|---|
3.5.2 | 2024年6月1日 |
#12 in 地理空间
78 每月下载量
用于 zero4rs
1MB
15K SLoC
google_maps
针对 Rust 编程语言的非官方 Google Maps Platform 客户端库。
此客户端当前实现了路线 API、距离矩阵 API、海拔 API、地理编码 API、时区 API,以及部分地点和道路 API。
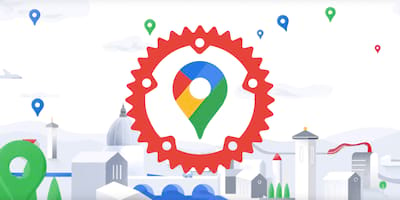
安装
配置依赖项
[dependencies]
google_maps = "3.5"
可选地,添加 rust_decimal = "1"
和 rust_decimal_macros = "1"
以访问 dec!
宏。此宏可以用于在您的程序中定义十进制数字。
这对于将纬度和经度硬编码到代码中进行测试很有用。
功能标志
可以通过功能标志单独启用所需的 Google Maps API。
此外,支持使用 rustls 对 Reqwest 进行查询。
Google Maps 客户端功能标志
自动完成
路线
距离矩阵
海拔
地理编码
地点
道路
时区
enable-reqwest
(使用 reqwest 查询 Google Maps API)。enable-reqwest-middleware
(使用 reqwest-middleware 查询 Google Maps API)。geo
(支持 geo crate 的类型)
注意:自动补全功能 autocomplete
包括与 Places API 自动补全相关的服务:地点自动补全请求 和 查询自动补全请求。所有其他 Places API 服务均由 places
功能覆盖。
reqwest 功能标志
仅适用于 enable-reqwest
。
native-tls
rustls
gzip
brotli
默认功能标志
默认情况下,Google Maps 客户端包括所有实现的 Google Maps API。Reqwest 将使用系统原生的 TLS (native-tls
) 加密连接,并启用 gzip 压缩 (gzip
)。
default = [
# Google Maps crate features:
"directions",
"distance_matrix",
"elevation",
"geocoding",
"time_zone",
"autocomplete",
"roads",
"places",
# reqwest features:
"enable-reqwest",
"reqwest/default-tls",
"reqwest/gzip",
# rust_decimal features:
"rust_decimal/serde",
]
功能标志使用示例
此示例将仅包括 Google Maps Directions API。Reqwest 将使用 Rustls 库加密连接,并启用 brotli 压缩。
google_maps = {
version = "3.5",
default-features = false,
features = [
"directions",
"enable-reqwest",
"rustls",
"brotli"
]
}
发行说明
完整的变更日志可在此处查看。
版本可在GitHub 上找到。
示例
路线 API
路线 API 是一种计算位置之间路线的服务。您可以搜索多种交通方式的路线,包括公共交通、驾车、步行或骑自行车。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let directions = google_maps_client.directions(
// Origin: Canadian Museum of Nature
Location::from_address("240 McLeod St, Ottawa, ON K2P 2R1"),
// Destination: Canada Science and Technology Museum
Location::try_from_f32(45.403_509, -75.618_904)?,
)
.with_travel_mode(TravelMode::Driving)
.execute()
.await?;
// Dump entire response:
println!("{:#?}", directions);
距离矩阵 API
距离矩阵 API 是一种提供起点和终点之间推荐路线的旅行距离和时间的矩阵服务。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let distance_matrix = google_maps_client.distance_matrix(
// Origins
vec![
// Microsoft
Waypoint::from_address("One Microsoft Way, Redmond, WA 98052, United States"),
// Cloudflare
Waypoint::from_address("101 Townsend St, San Francisco, CA 94107, United States"),
],
// Destinations
vec![
// Google
Waypoint::from_place_id("ChIJj61dQgK6j4AR4GeTYWZsKWw"),
// Mozilla
Waypoint::try_from_f32(37.387_316, -122.060_008)?,
],
).execute().await?;
// Dump entire response:
println!("{:#?}", distance_matrix);
高程 API (位置)
高程 API 为地球表面上的所有位置提供高程数据,包括海洋底部深度位置(返回负值)。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let elevation = google_maps_client.elevation()
// Denver, Colorado, the "Mile High City"
.for_positional_request(LatLng::try_from_dec(dec!(39.739_154), dec!(-104.984_703))?)
.execute()
.await?;
// Dump entire response:
println!("{:#?}", elevation);
// Display all results:
if let Some(results) = &elevation.results {
for result in results {
println!("Elevation: {} meters", result.elevation)
}
}
地理编码 API
地理编码 API 是一种提供地址地理编码和反向地理编码的服务。地理编码是将地址(如街道地址)转换为地理坐标(如纬度和经度)的过程,您可以使用这些坐标在地图上放置标记或将地图定位。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let location = google_maps_client.geocoding()
.with_address("10 Downing Street London")
.execute()
.await?;
// Dump entire response:
println!("{:#?}", location);
// Print latitude & longitude coordinates:
for result in location.results {
println!("{}", result.geometry.location)
}
反向地理编码 API
地理编码 API 是一种提供地址地理编码和反向地理编码的服务。反向地理编码是将地理坐标转换为可读地址的过程。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let location = google_maps_client.reverse_geocoding(
// 10 Downing St, Westminster, London
LatLng::try_from_dec(dec!(51.503_364), dec!(-0.127_625))?,
)
.with_result_type(PlaceType::StreetAddress)
.execute()
.await?;
// Dump entire response:
println!("{:#?}", location);
// Display all results:
for result in location.results {
println!(
"{}",
result.address_components.iter()
.map(|address_component| address_component.short_name.to_string())
.collect::<Vec<String>>()
.join(", ")
);
}
时区 API
时区 API 为地球表面上的位置提供时间偏移数据。您请求特定纬度/经度对和日期的时区信息。API 返回该时区的名称、与 UTC 的时间偏移以及夏令时偏移。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE");
// Example request:
let time_zone = google_maps_client.time_zone(
// St. Vitus Cathedral in Prague, Czechia
LatLng::try_from_dec(dec!(50.090_903), dec!(14.400_512))?,
// The time right now in UTC (Coordinated Universal Time)
Utc::now()
).execute().await?;
// Dump entire response:
println!("{:#?}", time_zone);
// Usage example:
println!("Time at your computer: {}", Local::now().to_rfc2822());
if let Some(time_zone_id) = time_zone.time_zone_id {
println!(
"Time in {}: {}",
time_zone_id.name(),
Utc::now().with_timezone(&time_zone_id).to_rfc2822()
);
}
地理定位 API
Google 的地理定位 API 似乎已离线。虽然在线文档仍然可用,并且通过 Google Cloud Platform 控制台似乎可以配置该 API,但地理定位 API 对所有请求都返回状态码 404 Not Found
和空内容。该 API 无法实现,直到服务器以预期方式响应。
控制请求设置
可以使用 Google Maps 客户端设置来更改请求速率和自动重试参数。
use google_maps::prelude::*;
let google_maps_client = GoogleMapsClient::new("YOUR_GOOGLE_API_KEY_HERE")
// For all Google Maps Platform APIs, the client will limit 2 sucessful
// requests for every 10 seconds:
.with_rate(Api::All, 2, std::time::Duration::from_secs(10))
// Returns the `GoogleMapsClient` struct to the caller. This struct is used
// to make Google Maps Platform requests.
.build();
反馈
我希望你在你的项目中取得成功!如果这个包不适合你,没有按照你的预期工作,或者如果你有请求或建议 - 请 向我报告!我可能不会立即回复,但我会回复。谢谢!
路线图
- 跟踪请求和请求元素以进行速率限制。
- 为此创建一个通用的
get()
函数,该函数可用于所有 API。 - 在合理的地方将显式查询验证转换为会话类型。
- 地点API。仅部分实现。如果您想实现任何缺失的部分,请与我联系。
- 道路API。仅部分实现。如果您想实现任何缺失的部分,请与我联系。
作者注
预计这个库可以很好地工作,并且实现了更多重要的谷歌地图功能。它应该会很好地工作,因为 serde 和默认的 reqwest 执行了大部分繁重的工作!
我创建了这个客户端库,因为我需要为我在做的项目中的几个谷歌地图平台功能。因此,我决定将我的库拆分为一个公开的crate。这是表示感激和回馈Rust社区的小小举动。希望它能节省某人的工作。
依赖项
~6–22MB
~358K SLoC