1 个不稳定版本
使用旧 Rust 2015
0.1.0 | 2015 年 12 月 2 日 |
---|
#60 在 #telegram-bot
6KB
103 行
此服务将于 2018 年 5 月 25 日关闭
由于许多原因,我们即将关闭此服务。您可以使用以下替代方案
- Chatbase — 来自 Google 的机器人分析,
- Dashbot,
- Botanalytics,
- Amplitude — 它有慷慨的免费计划,每月 10M 个事件。它是一个强大的分析工具,但没有针对机器人的特定功能。
关于此服务
Botan 是一个基于 Yandex.Appmetrica 的 Telegram 机器人分析系统。在此文档中,您可以找到如何设置 Yandex.Appmetrica 账户以及 Botan SDK 的使用示例。
Botan 有 2 个主要用例
- 将用户发送的每条消息的信息发送到 Botan,并获取基本使用统计信息,如 DAU、MAU、留存、命令等复杂细节。
- 通过包装您发送给他们的链接来获取关于用户的高级信息 — 您用户的位置、语言、设备和操作系统。
创建账户
- 在 http://appmetrica.yandex.com/ 注册
- 注册后,您将被提示创建应用程序。请使用 @YourBotName 作为名称。
- 从设置页面保存 API 密钥,您将使用它作为 Botan API 调用的令牌。
- 下载您语言的库,并按以下说明使用。不要忘记插入您的令牌!
SDK 使用
我们有以下语言的库
或者,您可以通过 纯 HTTP 调用 使用 Botan API。
如果您的首选语言缺失,您可以通过贡献来修复它。这很简单——库通常包含 30 行代码。
请注意 "要放入跟踪数据中的数据" 部分。90% 的分析使用优势在于正确的集成;)
JavaScript 示例
安装 npm: npm install botanio
var botan = require('botanio')(token);
botan.track(message, 'Start');
var uid = message.from.id;
var url = 'https://github.com/'; // some url you want to send to user
botan.shortenUrl(uid, url, function (err, res, body) {
if (err) {
console.error(err);
} else {
console.log(body); // shortened url here
}
});
Python 示例
要使用 Python Botan 库,您需要安装 requests 库。您可以使用以下方式完成此操作
pip install requests
代码
import botan
botan_token = '.........' # Token got from @botaniobot
uid = message.from_user
message_dict = message.to_dict()
event_name = update.message.text
print botan.track(botan_token, uid, message_dict, event_name)
.....
original_url = ... # some url you want to send to user
short_url = botan.shorten_url(original_url, botan_token, uid)
# now send short_url to user instead of original_url, and get geography, OS, Device of user
PHP 示例
您需要将类放在方便的位置。
private $token = 'token';
public function _incomingMessage($message_json) {
$messageObj = json_decode($message_json, true);
$messageData = $messageObj['message'];
$botan = new Botan($this->token);
$botan->track($messageData, 'Start');
...
$original_url = ...
$uid = $message['from']['id']
$short_url = $botan->shortenUrl($url, $uid)
// now send short_url to user instead of original_url, and get geography, OS, Device of user
}
Ruby 示例
uid
是从Telegram获取的用户ID。
require_relative 'botan'
token = 1111
uid = 1
message = { text: 'text' }
puts Botan.track(token, uid, message, 'Search')
Rust 示例
extern crate rustc_serialize;
extern crate botanio;
use botanio::{Botan};
#[derive(Debug, RustcEncodable)]
struct Message {
some_metric: u32,
another_metric: u32,
}
fn main() {
let token = "1111";
let uid = 1;
let name = "Search";
let message = Message {some_metric: 100, another_metric: 500};
let botan = Botan::new(token);
botan.track(uid, name, &message).unwrap();
}
Java 示例
try (CloseableHttpAsyncClient client = HttpAsyncClients.createDefault()) {
client.start();
Botan botan = new Botan(client, new ObjectMapper());
botan.track("1111", "1", ImmutableMap.of("some_metric": 100, "another_metric": 500), "Search").get();
}
Go 示例
package main
import (
"fmt"
"github.com/botanio/sdk/go"
)
type Message struct {
SomeMetric int
AnotherMetric int
}
func main() {
ch := make(chan bool) // Channel for synchronization
bot := botan.New("1111")
message := Message{100, 500}
// Asynchronous track example
bot.TrackAsync(1, message, "Search", func(ans botan.Answer, err []error) {
fmt.Printf("Asynchonous: %+v\n", ans)
ch <- true // Synchronization send
})
// Synchronous track example
ans, _ := bot.Track(1, message, "Search")
fmt.Printf("Synchronous: %+v\n", ans)
<-ch // Synchronization receive
}
Haskell 示例
import Network.HTTP.Client (newManager)
import Network.HTTP.Client.TLS (tlsManagerSettings)
import Servant.Client
import Web.Botan.Sdk
import GHC.Generics
import Data.Aeson
import Control.Concurrent.Async
main :: IO ()
main = do
manager <- runIO $ newManager tlsManagerSettings
let message = toJSON $ AnyMessage "A" "B"
a <- async $ track "token" "user2222" message "test_action" manager
res <- wait a -- not real use case
print res
data AnyMessage = AnyMessage
{
a :: Text
, b :: Text
} deriving (Show, Generic, ToJSON)
HTTP API
跟踪消息
基本URL是: https://api.botan.io/track
您应该使用POST方法将数据发送到Botan。
URL应如下所示 https://api.botan.io/track?token=API_KEY&uid=UID&name=EVENT_NAME
请提供一个JSON文档作为POST正文。
API响应是一个JSON文档
- 成功:{"status": "accepted"}
- 失败:{"status": "failed"} 或 {"status": "bad request", "info": "关于错误的某些附加信息"}
缩短URL
向以下地址发送GET请求
https://api.botan.io/s/?token={token}&url={original_url}&user_ids={user_id}
您将在纯文本响应中获得缩短的URL(如果响应代码为200)。除了200之外的其他代码表示发生了错误。
此外,在群聊的情况下,您还可以添加多个user_ids:&user_ids={user_id_1},{user_id_2},{user_id_3},但目前此数据将不会被使用(因为我们不知道哪个特定用户点击了链接)。
跟踪数据中应包含什么
###基本集成
botan.track(<botan_token>, <user_who_wrote_to_bot>, <user_message_in_json_format>, <command_name>)
- command_name - 我们建议在这里放置的不是消息文本,而是命令。例如:用户编写了'/search californication',将'Search'放入 command_name。这将帮助您聚合用户的输入类型并获取此类报告: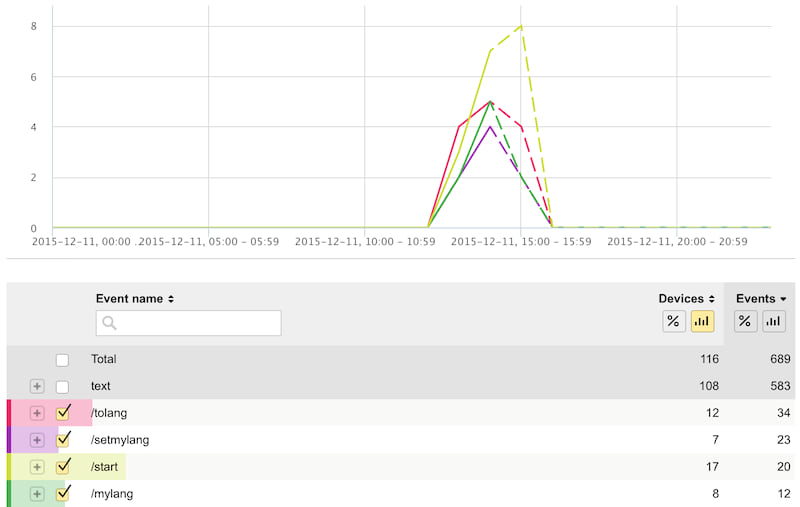
- user_message_in_json_format - 从Telegram获取的整个消息。例如,使用python-telegram-bot可以这样做:message.to_dict().传递整个消息,您将能够看到如下所示的数据:。您还可以获取执行特定操作的用户ID(通过分割)或最活跃的用户并联系他们: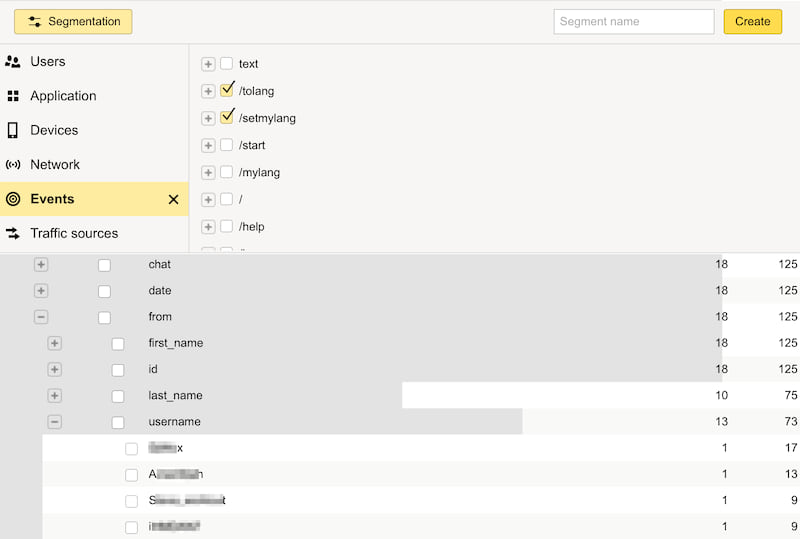
###高级集成 实际上,从分析中获取的最大好处在于发送正确的带正确数据的正确事件。以下是我们在最佳实践中推荐的一些方法。请随时贡献您的方法或改进现有方法。
#####命令顺序 这是您可以看到用户执行命令的顺序
botan.track(<botan_token>, <user_who_wrote_to_bot>, {last_command: current_command}, "command_order")
您还可以发送命令的三元组,而不是成对发送
botan.track(<botan_token>, <user_who_wrote_to_bot>, {before_last_command: {last_command: current_command}}, "command_order")
使用此方法,我们可以看到,例如,用户在执行/start命令后的命令: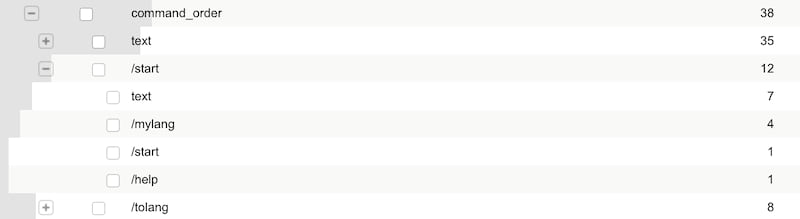
if this_is_first_occurence_of_user:
botan.track(<botan_token>,
<user_who_wrote_to_bot>,
{
'daily': message.date.strftime('%Y-%m-%d'),
'weekly': (message.date - datetime.timedelta(message.date.weekday())).strftime('%Y-%m-%d'),
'monthly': message.date.strftime('%Y-%m')
},
'cohorts')
#####时间群体 这是您如何根据用户在您服务中的第一天标记每个用户
### 工作原理 您为每对(用户,链接)创建一个独特的短链接。当用户点击链接时,Botan会存储他的用户代理、IP地址和其他信息。之后,您可以通过地理位置、语言、设备和操作系统进行用户细分(并查看相应的统计数据)。
### 要包装的url 我们建议您包装您发送给用户的每个url。最常见的用例是发送“请给我们评分”的链接——大多数流行的机器人都在storebot.me请求评分。
### 您将获得什么 您将在网络界面中获得大量有用的新数据
国家、地区和城市
设备
操作系统
区域
### 如何使用 在这里您可以找到Python和PHP的示例。请随意提交其他语言的包装器的pull请求(这里提供了短链接的HTTP规范)。
## 贡献 我们欢迎任何形式的pull请求!
请随意为我们的支持语言编写更多库。
lib.rs
:
Botan是基于Yandex.Appmetrica的Telegram机器人分析系统。
创建账户
在http://appmetrica.yandex.com/注册。
注册后,您将被提示创建应用程序。请使用 @YourBotName 作为名称。
从设置页面保存API密钥,您将使用它作为Botan
初始化的令牌。
有关更多信息,请参阅示例。
依赖关系
~5MB
~95K SLoC